算术表达式求值演示cpp
时间: 2024-10-27 09:01:36 浏览: 17
在C++中,计算算术表达式的求值通常涉及解析表达式、处理运算符优先级和结合性。这里我们可以使用递归下降解析器或库函数如`std:: stringstream` 来实现。下面是一个简单的递归函数来求解基本的加减乘除表达式:
```cpp
#include <iostream>
#include <string>
// 定义操作数和操作符类型
enum class Operator { Plus, Minus, Times, Divide };
int evaluate(std::string token, int numLeft = 0) {
if (token.empty()) return numLeft;
else if (isdigit(token[0])) { // 如果是数字,直接返回对应的数值
int numRight = std::stoi(token);
return numRight + numLeft; // 之前的数值会默认加在左边
}
else { // 否则处理运算符
char op = token[0];
int numRight = evaluate(token.substr(1), numLeft); // 递归处理剩余部分
switch (op) {
case '+':
return numRight + numLeft;
case '-':
return numRight - numLeft;
case '*':
return numRight * numLeft;
case '/':
return numRight / numLeft; // 注意除法可能引发除零错误
default:
throw std::runtime_error("Invalid operator");
}
}
}
int main() {
try {
std::string expression = "2 + 3 * 4";
int result = evaluate(expression);
std::cout << "Result of expression: " << result << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
阅读全文
相关推荐


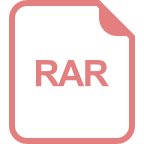









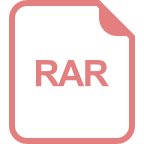
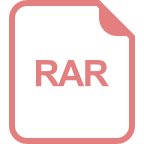



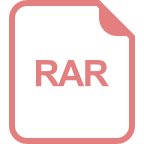
