用c语言写上面的代码
时间: 2024-11-10 07:25:12 浏览: 9
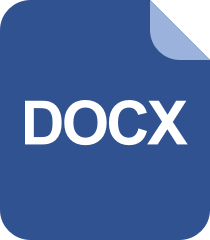
c语言文件读写操作代码
在C语言中,由于缺乏内置的数据结构如`Counter`,我们需要手动实现词频计数。这里是一个简单的示例,使用哈希表(数组+链表结构)来存储单词及其计数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义单词计数结构
typedef struct {
char* word;
int count;
} WordCount;
// 创建一个新的WordCount
WordCount* new_word_count(char* word) {
WordCount* wc = (WordCount*)malloc(sizeof(WordCount));
wc->word = strdup(word);
wc->count = 0;
return wc;
}
// 更新计数
void update_count(WordCount** counts, size_t count_index, char* word) {
if(counts[count_index] == NULL) {
counts[count_index] = new_word_count(word);
} else {
counts[count_index]->count++;
}
}
// 分析文本并打印词频
void analyze_text(const char* text) {
size_t word_count = 0;
WordCount* counts[1000]; // 假设最多有1000个不同的单词
// 清洗文本
char* cleaned_text = malloc(strlen(text) + 1);
strcpy(cleaned_text, text);
for(size_t i = 0; i < strlen(text); ++i) {
if(ispunct(cleaned_text[i])) {
cleaned_text[i] = ' ';
}
}
cleaned_text[strlen(text)] = '\0';
// 分割单词并更新计数
char* token = strtok(cleaned_text, " ");
while(token != NULL) {
update_count(counts, word_count++, token);
token = strtok(NULL, " ");
}
// 打印结果
printf("单词及其出现次数(降序):\n");
for(size_t i = 0; i < word_count; ++i) {
if(counts[i] != NULL) {
printf("%s: %d\n", counts[i]->word, counts[i]->count);
}
}
// 清理内存
for(size_t i = 0; i < word_count; ++i) {
free(counts[i]->word);
free(counts[i]);
}
free(cleaned_text);
}
int main() {
const char* example_text = "This is a sample text for word frequency analysis. It contains several words that will be counted.";
analyze_text(example_text);
return 0;
}
```
这个C语言版本的程序同样清洗了文本、分割单词并统计词频,不过因为C语言本身特性,需要手动管理内存分配和释放。
阅读全文
相关推荐
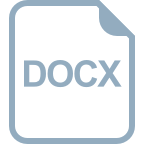
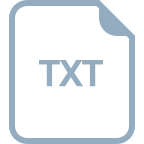















