c++ for循环同时遍历两个list
时间: 2023-09-18 22:06:59 浏览: 128
在C++中,可以使用`for`循环同时遍历两个`list`。假设要同时遍历`list1`和`list2`,可以按照以下方式进行:
```cpp
#include <iostream>
#include <list>
int main() {
std::list<int> list1 = {1, 2, 3, 4, 5};
std::list<int> list2 = {6, 7, 8, 9, 10};
// 获取两个list中较小的size
size_t size = std::min(list1.size(), list2.size());
// 使用迭代器进行遍历
auto it1 = list1.begin();
auto it2 = list2.begin();
for (size_t i = 0; i < size; ++i) {
// 输出对应位置的元素
std::cout << *it1 << " " << *it2 << std::endl;
// 迭代器向后移动
++it1;
++it2;
}
return 0;
}
```
上述代码中,通过使用两个迭代器`it1`和`it2`,在`for`循环中同时遍历了`list1`和`list2`。注意,在遍历过程中需要确保两个`list`的大小相同或者至少有相同数量的元素,否则可能会导致访问越界。
相关问题
写出c++中list容器遍历的方式
在C语言中没有标准的list容器,但可以使用链表来实现类似的功能。遍历链表的方式通常有两种:
1. 使用while循环遍历链表中的每个节点,直到遍历完整个链表。
```
struct ListNode {
int val;
struct ListNode *next;
};
void traverseList(struct ListNode* head) {
struct ListNode* current = head;
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
}
```
2. 使用for循环遍历链表中的每个节点,直到遍历完整个链表。
```
struct ListNode {
int val;
struct ListNode *next;
};
void traverseList(struct ListNode* head) {
for (struct ListNode* current = head; current != NULL; current = current->next) {
printf("%d ", current->val);
}
}
```
c++增强for循环
C++中没有像Java或Python中的增强for循环(foreach循环)那样的语法。然而,你可以使用迭代器或范围-based for循环来达到类似的效果。
一种常见的方法是使用迭代器来遍历容器中的元素。例如,对于一个std::vector<int>容器,你可以使用以下代码:
```cpp
std::vector<int> vec = {1, 2, 3, 4, 5};
for (std::vector<int>::iterator it = vec.begin(); it != vec.end(); ++it) {
int element = *it;
// 对每个元素执行操作
// ...
}
```
另一种方法是使用范围-based for循环,这是从C++11开始引入的一种更简洁的语法。使用范围-based for循环,你可以直接遍历容器中的元素,而不需要使用迭代器。以下是使用范围-based for循环的示例:
```cpp
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int element : vec) {
// 对每个元素执行操作
// ...
}
```
这两种方法都可以用于遍历各种容器,如std::vector、std::list、std::set等。记住,对于使用范围-based for循环,你无法修改容器中的元素,因为它会创建元素的副本。如果你需要修改容器中的元素,仍然需要使用迭代器。
相关推荐
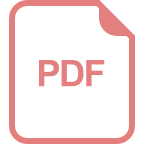
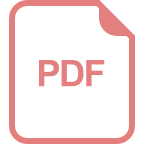
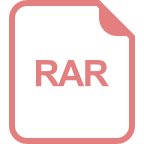












