请用【java】帮我写一段快排代码,并帮我讲解下实现逻辑定义Teacher类,包括姓名、年龄、性别三个成员变量,成员方法teach(),返回值类型为void,要求如下:① 属性私有,提供公开的get、set方法进行封装 ②提供无参数、有参数的构造方法 ③创建一个教师对象
时间: 2024-10-27 12:02:23 浏览: 34
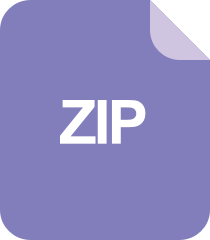
java代码-定义一个学生类
以下是使用Java编写的快速排序算法以及 Teacher 类的实现:
```java
// 快速排序算法
public class QuickSort {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j] <= pivot) {
i++;
swap(arr, i, j);
}
}
swap(arr, i + 1, high); // 将pivot放在正确的位置
return i + 1;
}
private static void swap(int[] arr, int i, int j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// Teacher 类
class Teacher {
private String name;
private int age;
private String gender;
// 构造方法
public Teacher() {
this.name = "";
this.age = 0;
this.gender = "";
}
public Teacher(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
// getter and setter methods
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
// teach method
public void teach() {
System.out.println("Teaching with " + name + ", a " + gender + " teacher who is " + age + " years old.");
}
}
// 创建教师对象并演示快速排序
public class Main {
public static void main(String[] args) {
Teacher teacher = new Teacher("张三", 35, "男");
teacher.teach();
// 快速排序示例
int[] numbers = {9, 7, 5, 11, 12, 2, 14};
QuickSort.quickSort(numbers, 0, numbers.length - 1);
// 输出排序后的数组
System.out.println("Sorted array:");
for (int num : numbers) {
System.out.print(num + " ");
}
}
}
```
这个程序首先定义了一个快速排序算法,然后有一个 Teacher 类,包含属性和相应的getter和setter方法,以及一个 teach 方法。在 `main` 函数里,我们创建了一个 Teacher 对象并调用了 teach 方法,最后展示了快速排序的过程。
阅读全文
相关推荐
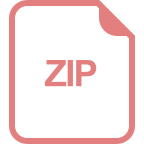
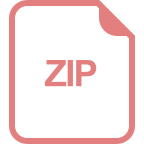
















