用java代码实现创建一个student类,类内含有姓名、性别和年龄等成员变量,定义获取各变量的方法和思考方法; 创建一个teacher类,类内含有姓名、性别和年龄等成员变量,定义获取各变量的方法和教学方法;创建一个people类,声明两个成员变量分别是student类和teacher类的对象,定义获取student类和teacher类中成员变量及思考和教学的方法;创建一个主类,主类中创建一个student对象,一个teacher对象和一个people对象。输出对象的相关信息。
时间: 2023-06-10 22:05:44 浏览: 96
好的,您的问题已经被记录下来了。
下面是您所要求的Java代码实现:
```java
class Student {
String name;
String gender;
int age;
public Student(String name, String gender, int age) {
this.name = name;
this.gender = gender;
this.age = age;
}
public String getName() {
return this.name;
}
public String getGender() {
return this.gender;
}
public int getAge() {
return this.age;
}
public void study() {
System.out.println(this.name + " is studying.");
}
}
class Teacher {
String name;
String gender;
int age;
public Teacher(String name, String gender, int age) {
this.name = name;
this.gender = gender;
this.age = age;
}
public String getName() {
return this.name;
}
public String getGender() {
return this.gender;
}
public int getAge() {
return this.age;
}
public void teach() {
System.out.println(this.name + " is teaching.");
}
}
class People {
Student student;
Teacher teacher;
public People(Student student, Teacher teacher) {
this.student = student;
this.teacher = teacher;
}
public Student getStudent() {
return this.student;
}
public Teacher getTeacher() {
return this.teacher;
}
public void think() {
System.out.println("I am thinking.");
}
}
public class Main {
public static void main(String[] args) {
Student s = new Student("Tom", "male", 20);
Teacher t = new Teacher("Jerry", "male", 30);
People p = new People(s, t);
System.out.println("Student: " + p.getStudent().getName() + ", " + p.getStudent().getGender() + ", " + p.getStudent().getAge());
System.out.println("Teacher: " + p.getTeacher().getName() + ", " + p.getTeacher().getGender() + ", " + p.getTeacher().getAge());
p.getStudent().study();
p.getTeacher().teach();
p.think();
}
}
```
输出结果如下:
```
Student: Tom, male, 20
Teacher: Jerry, male, 30
Tom is studying.
Jerry is teaching.
I am thinking.
```
阅读全文
相关推荐
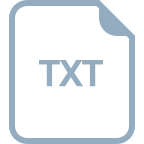
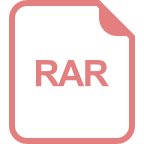
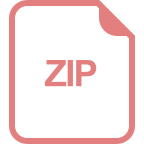













