给定一系列城市和每对城市之间的距离,求解访问每一座城市一次并回到起始城市的最短回路。相关代码
时间: 2024-10-08 16:17:58 浏览: 36
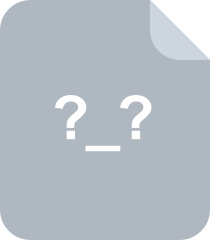
tsp_iter2.c
这个问题描述的是图论中的“旅行商问题”(Traveling Salesman Problem, TSP),它是一个经典的NP完全问题。在给定的距离矩阵表示的图中,每个节点代表一个城市,每条边代表两个城市之间的距离。目标是最优化地找到一条路径,使得销售员能访问所有城市恰好一次,并返回起点,总行程长度最小。
一种常见的启发式算法是“贪心策略”结合“最少费用生成树”(Minimum Spanning Tree, MST),例如“ Held-Karp 算法”。另一种较为复杂但更精确的方法是“动态规划”或使用一些近似算法,如“遗传算法”、“模拟退火”或者“蚁群算法”。
以下是使用 Python 和 `networkx` 库的一个简单示例,展示了如何使用 Kruskal's 或 Prim 算法先构建一个最小生成树,然后添加剩下的回环得到一个接近最优的解:
```python
import networkx as nx
# 假设 distances 是一个二维列表,存储城市之间的距离
distances = ...
# 创建无向加权图
graph = nx.Graph()
for i in range(len(distances)):
for j in range(i+1, len(distances[i])):
graph.add_edge(i, j, weight=distances[i][j])
# 使用 Kruskal's 或 Prim 算法创建最小生成树
mst = nx.minimum_spanning_tree(graph)
# 找到剩余的回路(从最后一个城市回到第一个)
last_city = list(mst)[-1]
path = [last_city]
current_city = last_city
while current_city != 0: # 起始城市通常是 0
path.append(current_city)
neighbors = mst.neighbors(current_city)
if neighbors:
shortest_neighbor = min(neighbors, key=lambda x: graph[current_city][x]['weight'])
current_city = shortest_neighbor
else:
break
path.append(0) # 添加回环到起始城市
# 最终路径
shortest_tsp_path = path[1:] # 删掉第一个(回程)
print("最短回路:", shortest_tsp_path)
```
请注意,这个解决方案只能提供近似的结果,而且对于大规模的真实世界问题,寻找精确解可能会很困难。
阅读全文
相关推荐
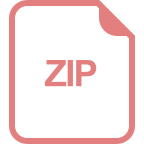
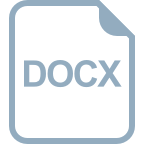


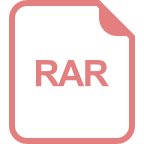
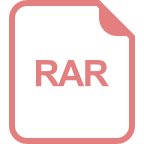
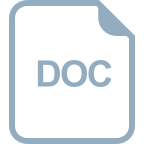
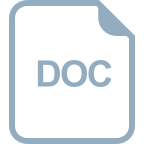
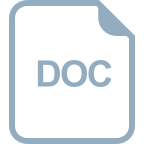
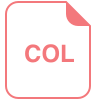
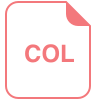
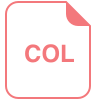
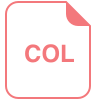
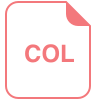
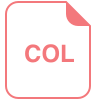


