C++编写的储存文件怎样放置到另一个文件里
时间: 2024-10-18 13:12:44 浏览: 13
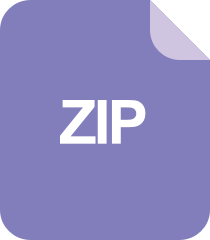
储存一些认真编写的C、C++语言代码.zip

在C++中,将文件内容从一个文件复制到另一个文件通常涉及两个步骤:首先读取源文件的内容,然后将其写入目标文件。以下是简单的步骤:
1. 打开源文件(输入模式,`ios::in`):
```cpp
std::ifstream inputFile("source_file.txt", std::ios::binary);
```
2. 检查文件是否成功打开,如果失败则处理错误。
3. 创建一个缓冲区来存储数据,或者直接读取到一个字符串或vector中:
```cpp
std::string content;
inputFile.seekg(0, std::ios::end); // 获取源文件长度
content.resize(inputFile.tellg());
inputFile.seekg(0, std::ios::beg); // 移回开始位置
inputFile.read(content.data(), content.size());
```
4. 关闭源文件。
5. 打开目标文件(输出模式,`ios::out`或`ios::trunc`若希望覆盖目标文件):
```cpp
std::ofstream outputFile("target_file.txt", std::ios::binary);
```
6. 写入数据到目标文件:
```cpp
outputFile.write(content.c_str(), content.size());
```
7. 最后记得关闭目标文件:
```cpp
outputFile.close();
```
阅读全文
相关推荐
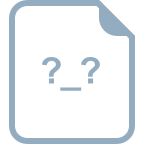
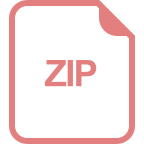

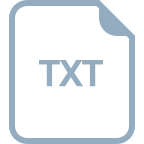
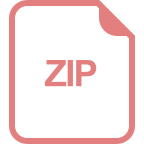
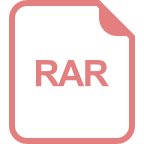
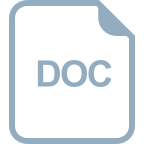










