C++在主线程中创建两个线程的示例
时间: 2024-09-24 08:15:49 浏览: 40
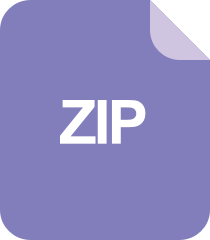
C++ 并发多线程日志处理
在C++中,你可以使用`std::thread`库来创建线程。下面是一个简单的例子,展示了如何在主线程中创建并启动两个独立的线程:
```cpp
#include <iostream>
#include <thread>
// 定义线程函数
void thread_function_1() {
std::cout << "Thread 1 is running." << std::endl;
// 线程的具体任务
for (int i = 0; i < 5; ++i) {
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Thread 1 iteration: " << i << std::endl;
}
}
void thread_function_2() {
std::cout << "Thread 2 is running." << std::endl;
// 同样的,这里是线程2的任务
for (int i = 0; i < 5; ++i) {
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "Thread 2 iteration: " << i << std::endl;
}
}
int main() {
// 创建线程1
std::thread t1(thread_function_1);
// 创建线程2
std::thread t2(thread_function_2);
// 等待线程完成(不是必需的,这里只是为了演示)
t1.join();
t2.join();
std::cout << "Both threads finished." << std::endl;
return 0;
}
```
在这个例子中,`main()`函数首先创建了两个`std::thread`对象`t1`和`t2`,并将它们分别绑定到`thread_function_1`和`thread_function_2`这两个线程函数上。然后,通过调用`join()`方法等待线程结束。
阅读全文
相关推荐
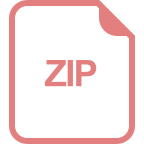
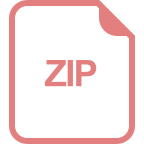
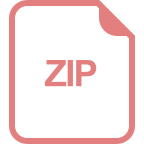
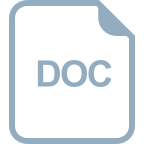













