python迷宫问题启发式搜索
时间: 2023-11-15 12:59:49 浏览: 125
Python是一种高级编程语言,它具有简单易学、代码可读性强、功能强大等特点,被广泛应用于Web开发、数据分析、人工智能等领域。
迷宫问题是指在一个迷宫中,从起点到终点的路径规划问题。启发式搜索是一种基于估价函数的搜索算法,它可以在搜索过程中尽可能地减少搜索空间,从而提高搜索效率。
在Python中,可以使用A*算法来解决迷宫问题。A*算法是一种启发式搜索算法,它通过估价函数来评估每个节点的优先级,从而选择最优的节点进行搜索。在迷宫问题中,估价函数可以是当前节点到终点的曼哈顿距离或欧几里得距离。
以下是Python实现A*算法解决迷宫问题的示例代码:
```python
import heapq
def astar(maze, start, end):
"""
A*算法解决迷宫问题
:param maze: 迷宫矩阵
:param start: 起点坐标
:param end: 终点坐标
:return: 路径列表
"""
# 定义估价函数
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
# 定义节点类
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0
self.h = 0
self.f = 0
def __lt__(self, other):
return self.f < other.f
# 初始化起点和终点节点
start_node = Node(start)
end_node = Node(end)
# 初始化开放列表和关闭列表
open_list = []
closed_list = set()
# 将起点节点加入开放列表
heapq.heappush(open_list, start_node)
# 开始搜索
while open_list:
# 取出开放列表中f值最小的节点
current_node = heapq.heappop(open_list)
# 如果当前节点是终点节点,返回路径
if current_node.position == end_node.position:
path = []
while current_node is not None:
path.append(current_node.position)
current_node = current_node.parent
return path[::-1]
# 将当前节点加入关闭列表
closed_list.add(current_node.position)
# 遍历当前节点的邻居节点
for next_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
# 计算邻居节点的坐标
neighbor_position = (current_node.position[0] + next_position[0], current_node.position[1] + next_position[1])
# 如果邻居节点不在迷宫范围内,跳过
if neighbor_position[0] < 0 or neighbor_position[0] >= len(maze) or neighbor_position[1] < 0 or neighbor_position[1] >= len(maze[0]):
continue
# 如果邻居节点是障碍物或已经在关闭列表中,跳过
if maze[neighbor_position[0]][neighbor_position[1]] == 1 or neighbor_position in closed_list:
continue
# 计算邻居节点的g值、h值和f值
neighbor_node = Node(neighbor_position, current_node)
neighbor_node.g = current_node.g + 1
neighbor_node.h = heuristic(neighbor_position, end_node.position)
neighbor_node.f = neighbor_node.g + neighbor_node.h
# 如果邻居节点已经在开放列表中,更新其f值和父节点
for open_node in open_list:
if neighbor_node.position == open_node.position and neighbor_node.f > open_node.f:
break
else:
# 将邻居节点加入开放列表
heapq.heappush(open_list, neighbor_node)
# 如果搜索失败,返回空路径
return []
```
阅读全文
相关推荐













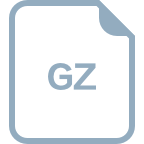




