python迷宫算法
时间: 2023-10-14 13:05:38 浏览: 151
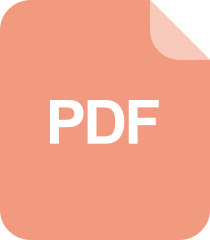
一文看懂Python迷宫生成和迷宫破解算法

a*算法是一种启发式搜索算法,用于解决迷宫寻路问题。它通过估计每个节点到目标节点的距离来选择下一个节点,从而尽可能地减少搜索的次数。在Python中,可以使用以下步骤实现a*算法:
1. 定义迷宫地图和起点、终点坐标。
2. 定义一个open列表和一个closed列表,分别用于存储待搜索和已搜索的节点。
3. 将起点加入open列表,并设置其f值为。
4. 重复以下步骤直到找到终点或open列表为空:
a. 从open列表中选取f值最小的节点作为当前节点。
b. 如果当前节点是终点,则返回路径。
c. 将当前节点从open列表中移除,并加入closed列表。
d. 遍历当前节点的相邻节点,如果相邻节点不在closed列表中,则计算其f值并加入open列表。
5. 如果open列表为空,则表示无法到达终点。
具体实现可以参考以下代码:
```python
import heapq
def a_star(maze, start, end):
# 定义节点类
class Node:
def __init__(self, x, y, g=, h=):
self.x = x
self.y = y
self.g = g # 起点到当前节点的距离
self.h = h # 当前节点到终点的估计距离
self.f = g + h # f = g + h
def __lt__(self, other):
return self.f < other.f
# 定义估价函数
def heuristic(node):
return abs(node.x - end[]) + abs(node.y - end[1])
# 初始化起点和终点节点
start_node = Node(start[], start[1])
end_node = Node(end[], end[1])
# 初始化open列表和closed列表
open_list = []
closed_list = set()
# 将起点加入open列表
heapq.heappush(open_list, start_node)
# 开始搜索
while open_list:
# 选取f值最小的节点作为当前节点
current_node = heapq.heappop(open_list)
# 如果当前节点是终点,则返回路径
if current_node.x == end_node.x and current_node.y == end_node.y:
path = []
while current_node:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
# 将当前节点加入closed列表
closed_list.add((current_node.x, current_node.y))
# 遍历当前节点的相邻节点
for dx, dy in [(, 1), (, -1), (1, ), (-1, )]:
x, y = current_node.x + dx, current_node.y + dy
if x < or x >= len(maze) or y < or y >= len(maze[]) or maze[x][y] == 1:
continue
if (x, y) in closed_list:
continue
# 计算相邻节点的f值
g = current_node.g + 1
h = heuristic(Node(x, y))
neighbor_node = Node(x, y, g, h)
neighbor_node.parent = current_node
# 如果相邻节点不在open列表中,则加入open列表
if neighbor_node not in open_list:
heapq.heappush(open_list, neighbor_node)
# 如果open列表为空,则表示无法到达终点
return None
```
其中,maze是一个二维数组,表示迷宫地图;start和end是起点和终点的坐标。迷宫地图中,表示可以通过的路径,1表示障碍物。返回的路径是一个坐标列表,从起点到终点。
阅读全文
相关推荐
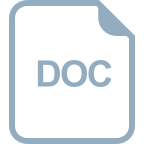
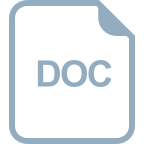





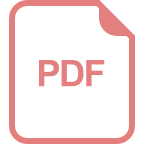



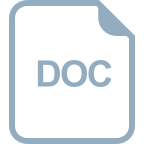
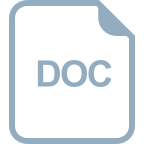
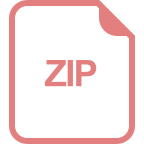