python 迷宫算法
时间: 2023-09-13 15:05:48 浏览: 77
迷宫算法可以使用深度优先搜索(DFS)和广度优先搜索(BFS)来解决。这里给出DFS的示例代码:
```python
def dfs_maze(maze, start, end):
"""
maze: 迷宫矩阵,0表示可行,1表示障碍
start: 起点坐标
end: 终点坐标
"""
rows, cols = len(maze), len(maze[0])
visited = [[False for _ in range(cols)] for _ in range(rows)]
path = []
dfs_helper(maze, start, end, visited, path)
return path
def dfs_helper(maze, curr, end, visited, path):
x, y = curr
if x < 0 or x >= len(maze) or y < 0 or y >= len(maze[0]) or visited[x][y] or maze[x][y] == 1:
return False
visited[x][y] = True
path.append(curr)
if curr == end:
return True
if dfs_helper(maze, (x-1, y), end, visited, path) or \
dfs_helper(maze, (x+1, y), end, visited, path) or \
dfs_helper(maze, (x, y-1), end, visited, path) or \
dfs_helper(maze, (x, y+1), end, visited, path):
return True
path.pop()
return False
```
其中,`dfs_maze`函数是迷宫算法的入口函数,`dfs_helper`函数是递归函数,用于实现DFS的搜索过程。`visited`矩阵用于记录某个坐标是否已经被访问过,`path`列表用于记录走过的路径。在`dfs_helper`函数中,首先判断当前坐标是否可行,若不可行则返回False,否则将当前坐标标记为已访问,并将其加入路径中。若当前坐标为终点,则返回True,否则分别向上、下、左、右四个方向递归调用`dfs_helper`函数,若其中一个方向返回True,则说明找到了一条路径,否则返回False,表示当前路径不可行。如果当前路径不可行,则需要将当前坐标从路径中删除,以便回溯到上一个可行坐标。
使用示例:
```python
maze = [[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]]
start = (0, 0)
end = (4, 4)
path = dfs_maze(maze, start, end)
print(path)
```
输出结果:
```
[(0, 0), (1, 0), (2, 0), (2, 1), (2, 2), (1, 2), (0, 2), (0, 3), (0, 4), (1, 4), (2, 4), (3, 4), (4, 4)]
```
可以看到,该算法找到了一条从起点到终点的路径。
阅读全文
相关推荐
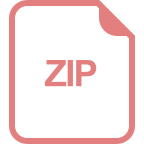

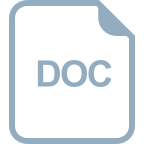







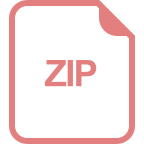