循环队列入队列和出队列的算法c语言实现
时间: 2024-06-22 09:02:26 浏览: 4
在C语言中,循环队列(Circular Queue)是一种特殊的线性表结构,它在队列的一端进行入队操作,在另一端进行出队操作,这样可以避免在队列满或空时移动元素的开销。以下是一个简单的循环队列的实现,包括入队(enqueue)、出队(dequeue)以及查看队列是否为空和满的方法:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 10 // 定义循环队列的最大容量
typedef struct {
int data[MAX_SIZE];
int front; // 前端指针
int rear; // 后端指针
} Queue;
// 初始化循环队列
void initQueue(Queue *q) {
q-1;
}
// 判断队列是否为空
bool isEmpty(Queue *q) {
return q->front == -1;
}
// 判断队列是否已满
bool isFull(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队操作
void enqueue(Queue *q, int value) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
if (isEmpty(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_SIZE; // 循环更新后端
}
q->data[q->rear] = value;
printf("Enqueued: %d\n", value);
}
// 出队操作
int dequeue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
int value = q->data[q->front];
if (q->front == q->rear) { // 队列只剩一个元素
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_SIZE; // 循环更新前端
}
printf("Dequeued: %d\n", value);
return value;
}
int main() {
Queue myQueue;
initQueue(&myQueue);
enqueue(&myQueue, 1);
enqueue(&myQueue, 2);
enqueue(&myQueue, 3);
dequeue(&myQueue);
return 0;
}
```
相关推荐
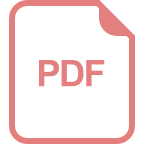
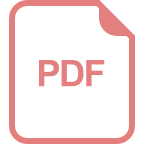














