C++编写一个简单的栈
时间: 2024-11-12 08:42:06 浏览: 4
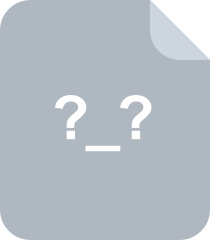
C++中用类编写简易的顺序栈
在C++中,我们可以使用数组或链表来创建一个基本的栈数据结构。这里以数组为基础的简单栈实现为例:
```cpp
#include <iostream>
const size_t MAX_STACK_SIZE = 10; // 定义栈的最大容量
// 定义栈的节点
struct StackElement {
int value;
bool is_top; // 标记当前元素是否为栈顶
};
class SimpleStack {
private:
StackElement stack[MAX_STACK_SIZE]; // 数组存储栈的数据
size_t top; // 栈顶指针,标记栈中最后一个元素的位置
public:
// 初始化栈
void init() {
top = -1;
}
// 判断栈是否已满
bool isFull() const {
return top == MAX_STACK_SIZE - 1;
}
// 入栈操作
void push(int value) {
if (isFull()) {
std::cout << "Stack overflow! Can't push to a full stack." << std::endl;
return;
}
stack[++top].value = value;
stack[top].is_top = true;
}
// 出栈操作
int pop() {
if (isEmpty()) {
std::cout << "Stack underflow! No element to pop." << std::endl;
return -1; // 返回无效值表示错误
}
StackElement temp = stack[top];
stack[top--] = {0, false}; // 清空栈顶元素
return temp.value;
}
// 获取栈顶元素但不移除(查看操作)
int peek() const {
if (isEmpty()) {
std::cout << "Stack is empty!" << std::endl;
return -1;
}
return stack[top].value;
}
};
int main() {
SimpleStack s;
s.init();
s.push(5);
s.push(10);
std::cout << "Top element: " << s.peek() << std::endl;
std::cout << "Popped element: " << s.pop() << std::endl;
return 0;
}
阅读全文
相关推荐
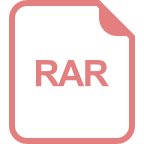
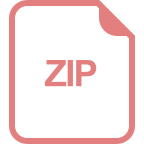
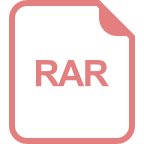




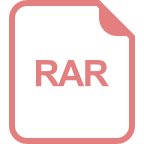
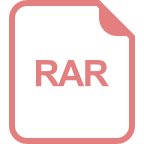
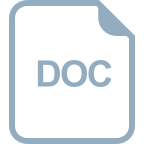
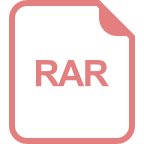
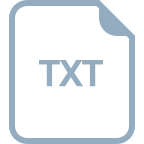
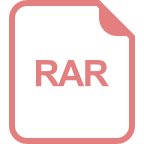
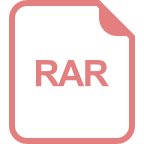
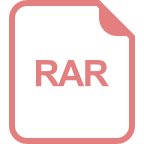
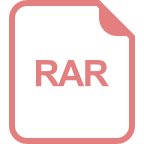

