编写C++代码遍历输出栈中所有元素
时间: 2024-10-21 19:14:49 浏览: 33
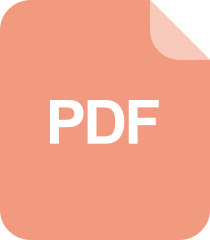
用C++实现一个链式栈的实例代码

在C++中,要遍历输出栈中的所有元素,你需要先创建一个栈,然后使用迭代器来访问和输出它们。这里是一个简单的例子,假设你使用`std::stack<int>`作为栈的数据结构:
```cpp
#include <iostream>
#include <stack>
// 创建一个包含整数的栈
std::stack<int> myStack = {10, 20, 30, 40, 50};
void printStack(const std::stack<int>& stack) {
// 使用top()函数获取栈顶元素,如果没有元素则出错
if (!stack.empty()) {
int topElement;
while (stack.top() != INT_MIN) { // INT_MIN是已知最小值,避免意外溢出导致错误
topElement = stack.top();
std::cout << "Top element is: " << topElement << std::endl;
// 出栈并继续循环
stack.pop();
}
} else {
std::cout << "The stack is empty." << std::endl;
}
}
int main() {
printStack(myStack);
return 0;
}
```
在这个示例中,`printStack`函数会从栈顶开始逐个取出元素并输出,直到栈变为空。
阅读全文
相关推荐
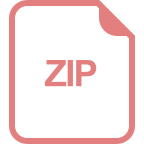
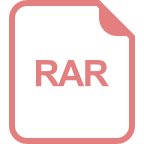
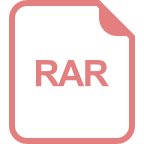
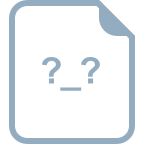
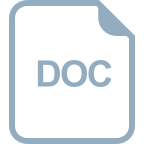
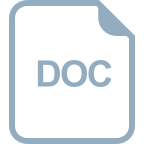
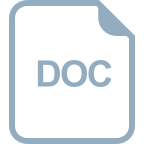
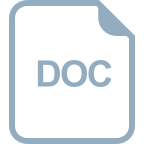
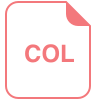







