设计一个集合类 Set,然后再编写一个应用程序,创建两个 Set 对象,并利 用 Math.random()产生这两个集合中的元素,然后对他们实施“交”、“并”操作。
时间: 2024-12-02 22:16:51 浏览: 2
设计一个集合类Set,通常我们选择使用Java的内置集合框架,如HashSet,因为Set不包含重复元素并且不允许有重复的元素插入。在Java中,我们可以这样做:
```java
import java.util.HashSet;
import java.util.Random;
// 集合类Set的示例
public class MyCustomSet<T> extends HashSet<T> {
// 如果需要自定义行为,可以在这里添加方法
}
public class Main {
public static void main(String[] args) {
// 创建两个MyCustomSet对象
MyCustomSet<Integer> set1 = new MyCustomSet<>();
MyCustomSet<Integer> set2 = new MyCustomSet<>();
// 使用Math.random()生成随机整数,并添加到集合中
Random random = new Random();
for (int i = 0; i < 10; i++) {
int num = random.nextInt(50); // 生成0-49之间的随机整数
set1.add(num);
set2.add(num);
}
// 打印初始集合
System.out.println("Set 1: " + set1);
System.out.println("Set 2: " + set2);
// 实现交集(Intersection)
MyCustomSet<Integer> intersection = new MyCustomSet<>(set1);
intersection.retainAll(set2); // 仅保留同时存在于两个集合的元素
// 打印交集
System.out.println("Intersection: " + intersection);
// 实现并集(Union)
MyCustomSet<Integer> union = new MyCustomSet<>(set1);
union.addAll(set2); // 将set2的所有元素添加到union中
// 打印并集
System.out.println("Union: " + union);
}
}
```
在这个例子中,我们首先创建了两个MyCustomSet对象,然后生成一些随机数添加到它们中。接着通过`retainAll()`方法实现了交集,而通过`addAll()`方法实现了并集。
阅读全文
相关推荐
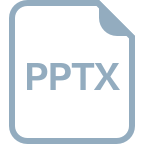
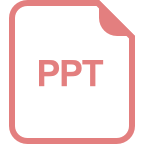
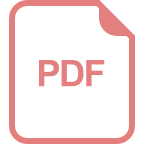
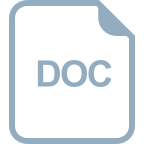
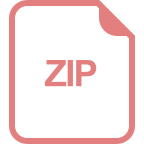
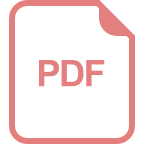
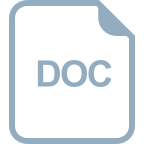
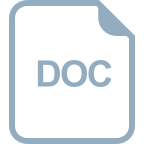
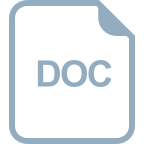
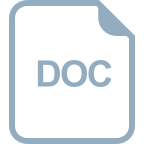
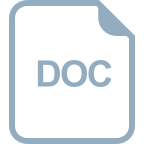
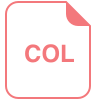
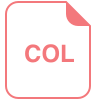





