定义Student学生类, 有id学号, name姓名, score成绩等属性定义List集合存储Student学生对象 向 List集合中添加 50 个Student对象, 要求id自增, 姓名随机生成, 成绩随机生成 要求使用stream流进行以下操作 1) 把集合中不及格同学的姓名保存到List集合中 2) 对list集合中的学生对象根据成绩降序排序, 如果成绩一样再根据学号升序排序, 把结果保存到Set集合中 3) 计算所有同学的平均分 4) 查看最高分同学 5) 查看最低分同学
时间: 2023-06-19 19:10:26 浏览: 207
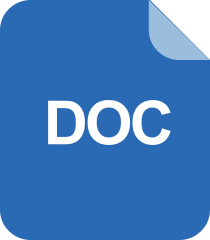
设计学生类Student,属性:编号(整型);姓名(字符串),成绩(整型)。

下面是Java代码实现:
```java
import java.util.*;
import java.util.stream.Collectors;
public class Student {
private int id;
private String name;
private int score;
public Student(int id, String name, int score) {
this.id = id;
this.name = name;
this.score = score;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
// 添加50个学生对象
for (int i = 1; i <= 50; i++) {
String name = getRandomName();
int score = (int) (Math.random() * 101);
Student student = new Student(i, name, score);
students.add(student);
}
// 1) 把集合中不及格同学的姓名保存到List集合中
List<String> failStudents = students.stream()
.filter(s -> s.getScore() < 60)
.map(Student::getName)
.collect(Collectors.toList());
System.out.println("不及格的学生有:" + failStudents);
// 2) 对list集合中的学生对象根据成绩降序排序, 如果成绩一样再根据学号升序排序, 把结果保存到Set集合中
Set<Student> sortedStudents = students.stream()
.sorted(Comparator.comparing(Student::getScore).reversed()
.thenComparing(Student::getId))
.collect(Collectors.toCollection(LinkedHashSet::new));
System.out.println("按照成绩排序后的学生有:" + sortedStudents);
// 3) 计算所有同学的平均分
double averageScore = students.stream()
.mapToInt(Student::getScore)
.average()
.orElse(0.0);
System.out.println("所有同学的平均分为:" + averageScore);
// 4) 查看最高分同学
Optional<Student> highestScoreStudent = students.stream()
.max(Comparator.comparing(Student::getScore));
if (highestScoreStudent.isPresent()) {
System.out.println("最高分同学是:" + highestScoreStudent.get().getName() + ",分数为:" + highestScoreStudent.get().getScore());
}
// 5) 查看最低分同学
Optional<Student> lowestScoreStudent = students.stream()
.min(Comparator.comparing(Student::getScore));
if (lowestScoreStudent.isPresent()) {
System.out.println("最低分同学是:" + lowestScoreStudent.get().getName() + ",分数为:" + lowestScoreStudent.get().getScore());
}
}
private static String getRandomName() {
String[] firstName = {"张", "王", "李", "赵", "刘", "陈", "杨", "黄", "吴", "周", "徐", "孙", "马", "朱", "胡", "林", "郭", "何", "高", "罗"};
String[] lastName = {"明", "红", "华", "强", "丽", "超", "涛", "艳", "峰", "秀", "勇", "磊", "莉", "军", "婷", "鹏", "萍", "伟", "敏", "飞"};
return firstName[(int) (Math.random() * firstName.length)] + lastName[(int) (Math.random() * lastName.length)];
}
}
```
阅读全文
相关推荐
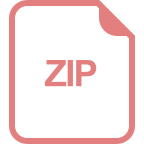
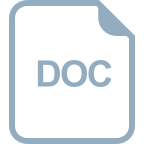








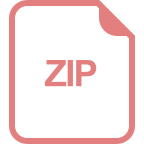
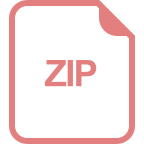
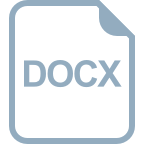
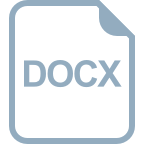