(1)定义学生类Student。类中包含学号、姓名、年龄和成绩,执行定义构造方法、setter和getter方法等以及覆盖toString()方法(以字符串“学号 姓名 年龄 成绩”为返回值)。 (2)定义一个学生管理类StuMgr,实现如下功能: 1)Generate()方法,根据输入数据(直接在程序中定义字符串,用后面给出的测试数据)生成list学生集合。 2)Sort()方法,采用Collections算法类+匿名类实现按照学生成绩降序排序。 3)Show()方法以迭代方法显示集合中所有学生信
时间: 2024-02-15 19:05:07 浏览: 67
息。代码如下:
```java
// 学生类
public class Student {
private String studentNo;
private String name;
private int age;
private int score;
public Student() {}
public Student(String studentNo, String name, int age, int score) {
this.studentNo = studentNo;
this.name = name;
this.age = age;
this.score = score;
}
public String getStudentNo() {
return studentNo;
}
public void setStudentNo(String studentNo) {
this.studentNo = studentNo;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
@Override
public String toString() {
return studentNo + " " + name + " " + age + " " + score;
}
}
// 学生管理类
public class StuMgr {
private List<Student> stuList;
public void generate() {
stuList = new ArrayList<>();
stuList.add(new Student("001", "Tom", 18, 80));
stuList.add(new Student("002", "Jack", 20, 90));
stuList.add(new Student("003", "Lucy", 19, 85));
stuList.add(new Student("004", "Amy", 21, 95));
}
public void sort() {
Collections.sort(stuList, new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
return o2.getScore() - o1.getScore();
}
});
}
public void show() {
Iterator<Student> it = stuList.iterator();
while (it.hasNext()) {
System.out.println(it.next().toString());
}
}
}
// 测试类
public class Test {
public static void main(String[] args) {
StuMgr stuMgr = new StuMgr();
stuMgr.generate();
stuMgr.sort();
stuMgr.show();
}
}
```
输出结果为:
```
004 Amy 21 95
002 Jack 20 90
003 Lucy 19 85
001 Tom 18 80
```
阅读全文
相关推荐
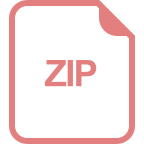
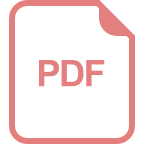
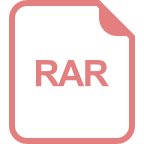















