使用链表完成20个学生信息的存储并顺序打印输出所有学生信息
时间: 2024-09-10 18:20:37 浏览: 60
使用链表存储和顺序打印20个学生信息的基本步骤如下:
1. 定义学生信息的数据结构:首先需要定义一个学生类,包含学生的姓名、年龄、学号等信息。
```java
class Student {
String name;
int age;
String studentNumber;
// 构造函数、getter和setter省略
}
```
2. 定义链表节点类:链表的节点通常包含数据和指向下一个节点的引用。在这里,节点的数据部分是学生信息。
```java
class StudentNode {
Student student;
StudentNode next;
StudentNode(Student student) {
this.student = student;
this.next = null;
}
}
```
3. 实现链表类:创建一个链表类,包含添加节点和打印链表的方法。
```java
class StudentLinkedList {
private StudentNode head;
public void addStudent(Student student) {
StudentNode newNode = new StudentNode(student);
if (head == null) {
head = newNode;
} else {
StudentNode current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void printAllStudents() {
StudentNode current = head;
while (current != null) {
System.out.println("Name: " + current.student.name + ", Age: " + current.student.age + ", Student Number: " + current.student.studentNumber);
current = current.next;
}
}
}
```
4. 创建链表实例并添加学生信息:创建一个`StudentLinkedList`的实例,并使用循环添加20个学生的信息。
```java
public class Main {
public static void main(String[] args) {
StudentLinkedList list = new StudentLinkedList();
for (int i = 1; i <= 20; i++) {
Student student = new Student("Student" + i, 20 + i, "S" + i);
list.addStudent(student);
}
list.printAllStudents();
}
}
```
以上代码演示了如何使用链表来存储学生信息,并在最后遍历链表打印出所有学生的信息。需要注意的是,这里的`Student`类、`StudentNode`类和`StudentLinkedList`类是简化版本,实际使用时可能需要添加构造函数、getter和setter方法等。
阅读全文
相关推荐
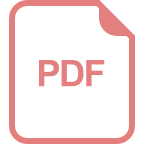
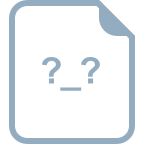
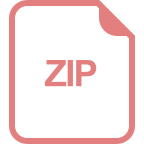




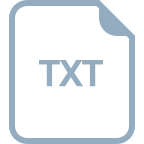
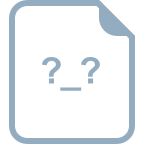
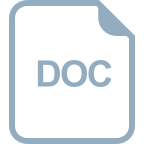
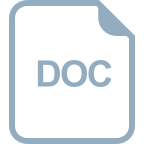
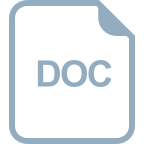
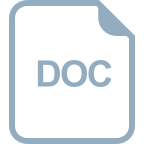




