定义学生结构体,使用单链表完成10个学生信息的存储并顺序打印输出所有学生信息,然后删除第6个学生的信息,并再次顺序打印输出所有学生信息
时间: 2024-09-19 15:02:53 浏览: 30
首先,我们来定义一个简单的`Student`结构体,包含姓名和年龄两个属性:
```c
struct Student {
char name[50];
int age;
struct Student* next; // 指向下一个学生的指针
};
```
接着,我们可以创建一个动态数组来表示10个学生的单链表。这里我们假设`createStudentList`函数用于创建和初始化这个列表:
```c
void createStudentList(struct Student** list) {
*list = (struct Student*)malloc(sizeof(struct Student));
for (int i = 0; i < 10; i++) {
(*list)[i].name = "Student" + i;
(*list)[i].age = i + 18;
(*list)[i].next = NULL;
if (i > 0) { // 链接前一个元素到当前元素
((*list)[i - 1]).next = &(*list)[i];
}
}
}
```
现在我们有10个学生信息存储在链表中了。要顺序打印所有学生信息,可以遍历链表并打印:
```c
void printStudentList(struct Student* list) {
while (list != NULL) {
printf("Name: %s, Age: %d\n", list->name, list->age);
list = list->next;
}
}
// 删除第6个学生信息
void deleteStudent(struct Student** list) {
if (*list == NULL || (*list)->next == NULL) return; // 如果链表为空或只有一个节点
struct Student* current = *list;
struct Student* prev = *list;
while (current != NULL && current->next != NULL && current->next->index != 5) {
prev = current;
current = current->next;
}
if (current != NULL) {
prev->next = current->next;
free(current); // 释放第6个学生的内存
}
}
// 再次打印学生列表
void printAfterDeletion(struct Student* list) {
printStudentList(list);
}
```
最后,你可以通过`createStudentList`, `printStudentList`, 和 `deleteStudent` 函数来完成整个操作。记得在程序结束时添加适当的内存清理步骤。
阅读全文
相关推荐
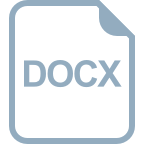
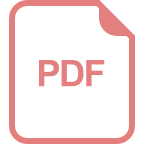
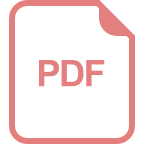

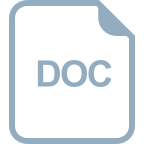

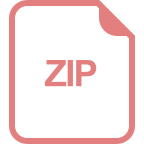
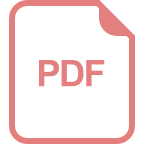
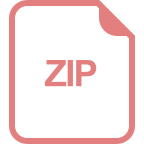
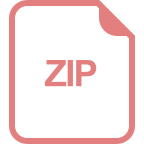
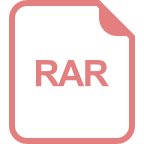
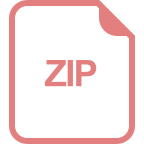
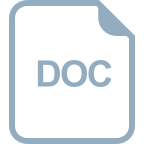
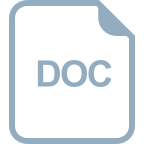




