设计一个算法,输出带头结点的非空单链表的第i个元素,若没有找到,输出不存在该元素的标志,C语言实现
时间: 2024-09-19 15:03:58 浏览: 47
在C语言中,设计一个遍历单链表寻找第i个元素的函数可以分为以下几个步骤:
1. 定义头节点结构体(Node),包含一个整数data和指向下一个节点的指针next。
2. 创建一个函数`get_nth_element`,接受链表头节点的指针`head`和目标索引`i`作为参数。
3. 初始化两个指针`current`和`previous`,将`current`设为头节点,`previous`设为空。
4. 使用循环从头开始遍历,直到找到第i个元素或遍历完整个链表。
5. 在每次迭代中,如果`current`不为空,检查它的data是否等于目标值,如果是,则返回当前节点的数据;同时更新`previous`为`current`,因为我们需要找到的是前一个节点(由于链表顺序存储)。
6. 如果达到链表尾部还未找到第i个元素,或者`current`为NULL(链表结束),则说明不存在目标元素,返回一个特殊的值(如-1或nullptr)表示不存在。
下面是C语言的实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
typedef struct Node {
int data;
struct Node* next;
} Node;
// 函数:获取链表第i个元素
int get_nth_element(Node* head, int i) {
if (head == NULL || i <= 0) return -1; // 检查输入有效性
Node* current = head;
Node* previous = NULL;
int count = 1;
while (current != NULL && count < i) {
previous = current;
current = current->next;
count++;
}
if (current == NULL) return -1; // 没有找到第i个元素
else return current->data;
}
// 测试代码
int main() {
// 创建并初始化链表...
Node* list_head = ...;
int index = 5; // 要查找的位置
int result = get_nth_element(list_head, index);
if (result == -1)
printf("Element at position %d does not exist.\n", index);
else
printf("The element at position %d is: %d\n", index, result);
return 0;
}
```
阅读全文
相关推荐
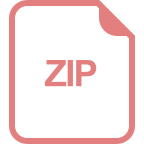
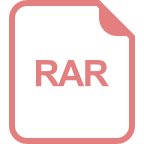
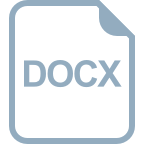















