设计一个算法,在带头结点的非空循环单链表L中的第一个最大值结点(最大值可能有多个)之前插入一个值为x的结点,使用c语言方法
时间: 2024-10-20 21:05:15 浏览: 0
在C语言中设计这样的算法,我们需要首先找到循环链表中的最大值节点,然后在其前面插入新节点。这里是一个简化的步骤:
1. **初始化变量**:
- 定义一个指针`current`,指向头节点`head`,并设置两个辅助指针`prev`和`maxNode`,用于遍历和保存最大值。
2. **查找最大值节点**:
- `prev = current;`
- `while (true)`:
- `if (current->value > maxNode->value)`:
- `maxNode = current;`
- 如果遇到`prev == current`,说明找到了循环,此时`maxNode`即为循环内的最大值节点。
- `prev = current->next;`
- `current = current->next;`
- 如果`current == prev`,回到头部开始,继续遍历直到找到循环。
3. **插入新节点**:
- 创建一个新的节点`newNode`,其值设为`x`。
- 如果`maxNode`不是头节点,那么`prev->next = newNode`;否则(即`head`就是最大值),`newNode->next = head->next`,然后`head->next->prev = newNode`,确保新节点连接到了正确的前驱节点。
- 最后,更新`head->next = newNode`,因为新节点成为了新的头节点之前的节点。
```c
struct Node* insertAfterMax(struct Node* head, int x) {
if (head == NULL) return head;
struct Node *current = head;
struct Node *prev = head;
struct Node *maxNode = head;
while (true) {
if (current->value > maxNode->value) {
maxNode = current;
}
if (prev == current) {
break;
}
prev = current->next;
current = current->next;
}
struct Node *newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->value = x;
newNode->next = maxNode->next;
if (maxNode->next != head) {
prev->next = newNode;
} else {
newNode->next->prev = newNode;
head->next = newNode;
}
return head;
}
```
阅读全文
相关推荐
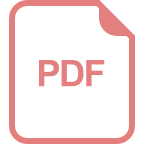
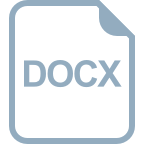
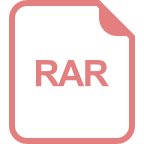















