用Python绘制海底地形图
时间: 2024-06-23 14:01:48 浏览: 373
在Python中绘制海底地形图通常会使用科学可视化库,如matplotlib和 cartopy,结合海洋数据(如SRTM数据或海洋格点数据)来进行。以下是一个简单的步骤概述:
1. 安装必要的库:首先,确保安装了`matplotlib`, `cartopy`, 和可能的数据处理库如`rasterio`和`geopandas`。
```bash
pip install matplotlib cartopy rasterio geopandas
```
2. 加载海洋数据:你可以从NASA的DEM(数字高程模型)数据源下载SRTM数据,如`srtm90`。使用`rasterio`读取这些数据。
3. 设置地图投影:由于海底地形图通常需要全球性的视角,所以可能需要使用`cartopy`库中的 PlateCarree 或 Mercator 等投影。
4. 绘制海底地形:将高程数据转换为等深线或者颜色编码的海拔图,使用`imshow`或`contourf`函数。
5. 添加海岸线和图例:使用`cartopy`的海岸线功能,加上合适的图例来解释颜色或等深线的含义。
6. 整体布局和保存:调整图像大小、添加标题、坐标轴标签,然后保存成图片文件。
下面是一个简化的代码示例,展示如何使用这些库:
```python
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
import rasterio
from cartopy.feature import ShapelyFeature
# 读取SRTM数据
with rasterio.open('srtm_data.tif') as src:
bathymetry = src.read(1)
# 创建地图对象
fig, ax = plt.subplots(
figsize=(10, 8), subplot_kw={'projection': ccrs.PlateCarree()})
# 设置等深线的颜色和宽度
cmap = 'Blues_r'
levels = np.arange(bathymetry.min(), bathymetry.max(), 100)
ax.coastlines(resolution='10m')
ax.add_feature(ShapelyFeature(src.bounds, ccrs.PlateCarree(), edgecolor='black'))
# 绘制海底地形
image = ax.imshow(bathymetry, cmap=cmap, transform=ccrs.PlateCarree(),
extent=src.bounds, levels=levels)
# 添加颜色bar
cbar = fig.colorbar(image, orientation='vertical', label='Depth (m)')
# 添加标题和坐标轴标签
ax.set_title('Underwater Topography Map')
ax.set_global()
# 保存图像
plt.savefig('submarine_topo_map.png')
```
阅读全文
相关推荐










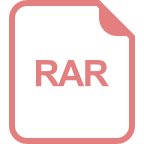


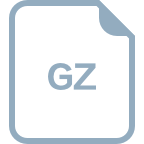


