用C++代码实现:设a[0:n-1]是已排好序的数组,请改写二分搜索算法,使得当搜索元素x不在数组中时,返回小于x的最大元素位置i和大于x的最小元素位置j:当搜索元素在数组中时,i和j相同,均为x在数组中的位置
时间: 2024-09-30 15:04:57 浏览: 32
在C++中,你可以通过一个稍微修改过的二分查找算法实现这个需求。这里有一个示例,首先定义一个函数`binarySearch`,它接受一个排序好的整数数组`arr`,长度`n`,以及我们要查找的目标值`x`。该函数将返回一个结构体`Result`,包含目标元素的位置`i`和小于`x`的最大元素位置`j`。
```cpp
#include <vector>
#include <utility>
// 结果结构体
struct Result {
int i; // x在数组中的位置
int j; // 小于x的最大元素位置
};
// 二分查找函数
Result binarySearch(std::vector<int>& arr, int n, int x) {
int left = 0;
int right = n - 1;
int i = -1; // 初始化目标元素位置
int max_j = -1; // 初始化小于x的最大元素位置
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == x) {
i = mid;
// 如果找到x并且还未找到最大小于x的元素,则更新max_j
if (max_j == -1 || arr[mid - 1] > x)
max_j = mid - 1;
break;
} else if (arr[mid] < x) {
left = mid + 1;
if (mid > 0 && arr[mid - 1] > x) // 更新最大小于x的元素位置
max_j = mid - 1;
} else { // arr[mid] > x
right = mid - 1;
}
}
Result result;
result.i = i;
result.j = max_j >= 0 ? max_j : n; // 当x不存在时,设置j为数组的最后一个元素
return result;
}
int main() {
std::vector<int> arr = {1, 3, 4, 6, 8, 9};
int n = arr.size();
int x = 5;
Result res = binarySearch(arr, n, x);
if (res.i != -1) {
std::cout << "Element found at index " << res.i << ". Smallest greater element is at index " << res.j << std::endl;
} else {
std::cout << "Element not found. Smallest greater element than " << x << " is at the end of array." << std::endl;
}
return 0;
}
```
在这个代码里,我们处理了两个情况:元素存在于数组中和元素不存在于数组中。如果元素存在,直接返回其索引;如果元素不存在,我们会找到小于它的最大元素的索引,并将其作为`j`的值返回。
阅读全文
相关推荐



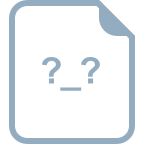
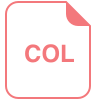
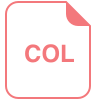
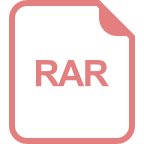
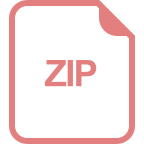
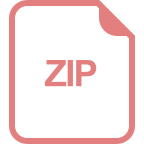
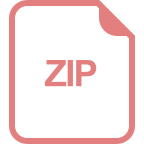
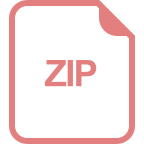
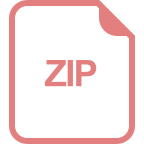