编写算法求二维数组中任一元素存储在一维内存单元中的位置。用C++方法
时间: 2024-11-16 13:19:43 浏览: 14
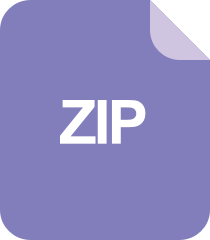
二维基本图形几何变换算法(用VC++实现)
为了将二维数组的任意元素存储在一维内存中,我们可以使用矩阵的行优先(Row Major)存储策略,即数组的索引按照先列后行的方式生成。假设我们有一个二维整型数组`arr`,其大小为`m`行`n`列,可以创建一个新的一维数组`flatArr`,然后通过计算每个元素的偏移量将其插入到这个一维数组中。
下面是一个简单的C++示例:
```cpp
#include <iostream>
// 定义二维数组
int arr[m][n];
// 计算二维数组元素的一维索引
int calculateIndex(int row, int col) {
return row * n + col; // 行优先存储,索引 = (行 * 列数) + 列
}
int main() {
// 初始化二维数组
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
arr[i][j] = some_value; // 假设这里有一个初始值
}
}
// 创建一维数组并填充
int flatSize = m * n;
int* flatArr = new int[flatSize];
for (int i = 0, index = 0; i < m && index < flatSize; i++, index++) {
flatArr[index] = arr[i][j]; // 这里假设你想复制整个行或列
}
// 现在flatArr包含了二维数组的所有元素
// 如果需要获取特定元素的位置,可以像下面这样使用calculateIndex函数
int targetValue = arr[4][7]; // 假设要查找的值
int position = calculateIndex(4, 7); // 获取该值在一维数组中的位置
delete[] flatArr; // 别忘了释放一维数组的内存
std::cout << "The position of element " << targetValue << " is: " << position << std::endl;
return 0;
}
阅读全文
相关推荐
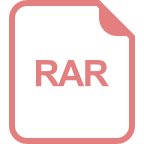
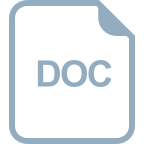
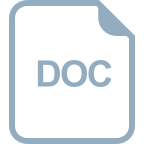

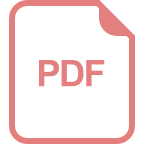
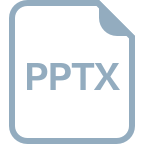
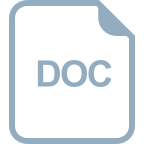
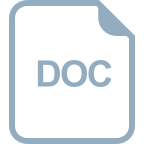
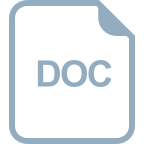
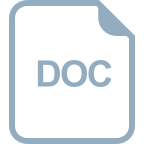
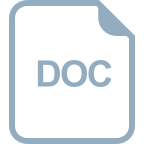
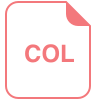
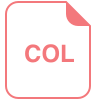
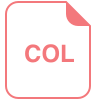


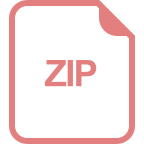