kotlin mvi
时间: 2024-05-29 15:06:50 浏览: 19
# Kotlin MVI
- Model
- 存储状态数据
- 提供访问和修改方法
- 将操作转为意图
- 订阅模型状态更新界面
- 转发用户操作给Presenter
- Intent
- 代表用户操作或系统事件
- 发送给Presenter处理
- Presenter
- 模型和视图之间的中间人
- 接收视图的意图并更新模型
- 可从外部数据源获取数据并更新模型
- 单向数据流动
- 用户操作发送意图到Presenter处理
- Presenter更新模型并发送给View展示
相关问题
kotlin实现mvi框架
MVI是一种基于响应式编程的架构模式,它可以帮助我们更有效地管理应用程序的状态和响应用户交互。在Kotlin中实现MVI框架需要以下步骤:
1. 定义状态类
首先,我们需要定义一个状态类,它将存储应用程序的状态。该类应该包含应用程序中所有可能的状态,例如:加载中、成功、错误等。
```kotlin
sealed class State {
object Loading : State()
data class Success(val data: List<String>) : State()
data class Error(val message: String) : State()
}
```
2. 定义Action类
然后,我们需要定义一个Action类,它将存储用户操作的信息。该类应该包含应用程序中所有可能的操作,例如:加载数据、刷新数据等。
```kotlin
sealed class Action {
object LoadData : Action()
object RefreshData : Action()
}
```
3. 定义Reducer函数
Reducer函数是MVI模式的核心。Reducer函数将当前状态和Action作为参数,并返回新状态。在Reducer函数中,我们可以根据Action来进行状态的更新。
```kotlin
fun reduce(state: State, action: Action): State {
return when (action) {
is Action.LoadData -> State.Loading
is Action.RefreshData -> state
}
}
```
4. 定义View层
View层是用户界面的部分,它将显示应用程序的状态和响应用户操作。在View层中,我们需要订阅状态的变化,并根据状态来更新用户界面。
```kotlin
class MyActivity : AppCompatActivity() {
private lateinit var viewModel: MyViewModel
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_my)
viewModel = ViewModelProvider(this).get(MyViewModel::class.java)
viewModel.state.observe(this, Observer { state ->
when (state) {
is State.Loading -> showLoading()
is State.Success -> showData(state.data)
is State.Error -> showError(state.message)
}
})
viewModel.dispatch(Action.LoadData)
}
private fun showLoading() {
// 显示加载中的UI
}
private fun showData(data: List<String>) {
// 显示数据的UI
}
private fun showError(message: String) {
// 显示错误的UI
}
}
```
5. 定义ViewModel层
ViewModel层是连接View层和Reducer函数的部分。在ViewModel层中,我们需要将Action传递给Reducer函数,并将新状态发送给View层。
```kotlin
class MyViewModel : ViewModel() {
private val _state = MutableLiveData<State>()
val state: LiveData<State> = _state
fun dispatch(action: Action) {
val newState = reduce(_state.value ?: State.Loading, action)
_state.postValue(newState)
}
}
```
这就是Kotlin中实现MVI框架的基本步骤。当然,在实际应用中,我们可能需要更复杂的状态、Action和Reducer函数,但是这些步骤应该是通用的。
MVI框架kotlin
MVI(Model-View-Intent)是一种架构模式,用于开发响应式和可预测的用户界面。它在Kotlin中得到广泛应用。
在MVI架构中,Model表示应用程序的状态和数据。View是用户界面的呈现层,负责显示数据和接收用户输入。Intent是由用户行为触发的动作,通常用于更新Model。
在Kotlin中,你可以使用许多开源框架来实现MVI架构。其中一些流行的选择包括:
1. RxJava:RxJava是一个响应式编程库,可以用于处理异步和事件驱动的数据流。它可以与MVI模式结合使用,通过Observables和观察者模式来管理状态和用户交互。
2. Kotlin Coroutines:Kotlin Coroutines是Kotlin官方提供的异步编程框架。它提供了一种简化异步操作的方式,并且可以与MVI架构很好地结合使用。
3. Mosby:Mosby是一个专门为Android应用程序设计的MVI框架。它提供了一套简单易用的API,帮助你在Android应用中实现MVI架构。
4. Mobius:Mobius是一个通用的函数响应式编程库,适用于Java和Kotlin。它提供了一个纯函数式的MVI框架,帮助你构建可测试和可扩展的应用程序。
这些都是一些常见的MVI框架选择,你可以根据自己的需求和偏好选择适合你的框架。
相关推荐
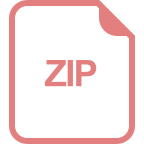
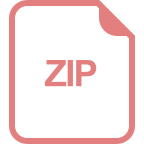
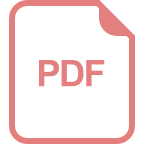












