pandas group by
时间: 2023-10-19 19:34:13 浏览: 42
Pandas的group by操作是一种用于对数据进行分组和聚合的功能。通过group by操作,我们可以按照指定的列或条件将数据分成不同的组,然后对每个组进行聚合计算。
在Pandas中,可以使用`groupby()`方法来实现group by操作。下面是一个示例:
```python
import pandas as pd
# 创建一个DataFrame
data = {'Name': ['Tom', 'Nick', 'John', 'Tom', 'John'],
'Score': [70, 80, 90, 60, 85],
'Subject': ['Math', 'English', 'Math', 'English', 'Math']}
df = pd.DataFrame(data)
# 按照Name列进行分组,计算每个学生的平均分
grouped_df = df.groupby('Name')['Score'].mean()
print(grouped_df)
```
以上代码中,我们创建了一个包含学生姓名、分数和科目的DataFrame。通过`groupby()`方法,我们将数据按照姓名进行分组,并计算每个学生的平均分。最后输出的结果是每个学生的平均分。
除了平均值,还可以使用其他聚合函数(如`sum()`、`count()`、`max()`、`min()`等)对每个组进行计算。
希望这个示例能够帮助你理解pandas中的group by操作。如果有更多问题,请随时提问!
相关问题
pandas groupby
### 回答1:
Pandas groupby 是一个非常强大的数据聚合工具,可以根据数据中的某些属性对数据进行分组,并按照分组后的标准进行聚合操作。常见的聚合操作包括计算平均值、求和、统计个数等等。下面是一个简单的示例代码,用于演示 Pandas groupby 的基本用法:
```
import pandas as pd
df = pd.read_csv('data.csv')
grouped = df.groupby(['category'])
result = grouped.agg({'price': ['mean', 'sum'], 'quantity': 'sum'})
print(result)
```
这段代码中,我们首先使用 Pandas 读取了一个 CSV 文件,并将其存储在 DataFrame 中。然后,我们对数据按照 'category' 属性进行分组,并计算了每个分组的平均价格、总价格和总数量。最后,我们将结果打印出来。
需要注意的是,Pandas groupby 还有很多高级用法,例如可以自定义聚合函数、使用多个属性进行分组、使用时间序列数据进行分组等等。如果你对 Pandas groupby 感兴趣,可以查看 Pandas 官方文档中的 Group By: split-apply-combine。
### 回答2:
pandas的groupby是一个强大的数据处理工具,可以对数据进行分组并进行各种操作。在使用groupby之前,需要先通过pandas库导入数据,并对数据进行处理。
首先,使用pandas的read_csv函数读取csv文件,并保存为一个DataFrame对象。然后,根据需要选择需要分组的列,并调用groupby函数。
groupby函数可以接收一个或多个分组的列名作为参数,将数据按照这些列进行分组。分组后,可以对每个组进行各种操作,比如计数、求和、平均值等等。
接下来,可以使用agg函数对分组后的数据进行聚合操作。agg函数可以接收一个或多个聚合函数作为参数,比如count、sum、mean等等。聚合函数将对每个组内的数据进行计算,并将结果返回为一个新的DataFrame对象。
除了agg函数,还可以使用transform函数对分组后的数据进行转换操作。transform函数可以接收一个或多个转换函数作为参数,并将转换后的结果与原数据对应,返回一个新的DataFrame对象。
最后,通过reset_index函数可以将分组后的结果重新索引,得到一个新的DataFrame对象。
总的来说,pandas的groupby是一个非常强大的工具,能够方便地对数据进行分组和聚合操作,提高数据处理和分析的效率。
### 回答3:
Pandas的groupby是一种基于某一或多个列对数据进行分组的操作。通过groupby可以将数据集分成若干个组,并对每个组应用相同的操作。
首先,我们需要使用groupby函数指定要分组的列。可以使用单个列名或多个列名作为groupby函数的参数。然后,我们可以对分组后的数据应用各种聚合函数,例如求和、平均值、计数等。
groupby返回的是一个GroupBy对象,这个对象包含了分组后的数据,以及一些可以进行聚合操作的方法和属性。
使用groupby时,常用的聚合操作之一是使用agg函数对分组后的数据进行多个不同的聚合操作。通过传递一个字典给agg函数,可以对每个聚合操作指定一个列名。
另外,groupby还具有分组过滤和转换的功能。分组过滤可以通过使用filter函数对分组后的数据进行筛选。分组转换可以通过使用transform函数对分组后的数据进行改变,但是保持数据形状的不变。
总而言之,Pandas的groupby是一种很方便的数据处理工具,它可以快速对数据进行分组,并进行各种聚合、过滤和转换操作。它在数据分析和处理中经常被使用到,能够提高数据分析的效率和准确性。
pandas groupby用法
pandas的groupby()方法是按照指定的列名或函数进行分组的操作。它可以用于数据聚合、数据处理和数据分析等场景。
下面是groupby()的基本用法:
1. 按列名分组:
```
df.groupby('列名')
```
2. 按多个列名分组:
```
df.groupby(['列名1','列名2'])
```
3. 按函数分组:
```
df.groupby(func)
```
其中,func是一个函数,它的返回值将作为分组依据。
4. 分组后进行聚合操作:
```
df.groupby('列名').agg(聚合函数)
```
其中,聚合函数可以是sum、mean、median、max、min等统计函数。
5. 分组后进行数据转换操作:
```
df.groupby('列名').transform(函数)
```
其中,函数可以是lambda函数或其他自定义函数。
6. 分组后进行数据过滤操作:
```
df.groupby('列名').filter(函数)
```
其中,函数返回一个布尔值,用于过滤数据。
这些是pandas groupby的基本用法,希望能帮到你。
相关推荐
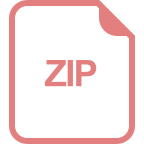
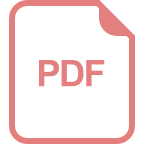








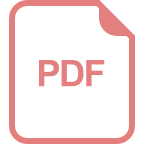
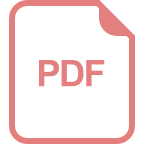