用python语言 读取图像,图像做灰度处理,并且分割矩阵,将图像分成上下左右四个图像,并显示
时间: 2024-10-07 09:01:45 浏览: 45
在Python中,我们可以使用PIL(Pillow)库来处理图像,NumPy进行数组操作,以及matplotlib库来显示图片。以下是分步骤的示例:
1. 首先,安装所需的库(如果还没有安装):
```bash
pip install pillow numpy matplotlib
```
2. 然后,你可以使用以下代码读取图像、转换为灰度并分割成四部分:
```python
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# 读取图像
image = Image.open('your_image.jpg') # 替换为你的图片路径
# 转换为灰度
gray_image = image.convert('L')
# 将图像转为numpy数组
gray_array = np.array(gray_image)
# 分割图片为四部分
height, width = gray_array.shape
top_left = gray_array[:height//2, :width//2]
top_right = gray_array[:height//2, width//2:]
bottom_left = gray_array[height//2:, :width//2]
bottom_right = gray_array[height//2:, width//2:]
# 显示四部分图像
fig, axs = plt.subplots(2, 2)
axs[0, 0].imshow(top_left, cmap='gray')
axs[0, 1].imshow(top_right, cmap='gray')
axs[1, 0].imshow(bottom_left, cmap='gray')
axs[1, 1].imshow(bottom_right, cmap='gray')
for ax in axs.flat:
ax.set(xticks=[], yticks=[]) # 移除坐标轴刻度
plt.show()
```
记得替换`'your_image.jpg'`为你实际的图像文件路径。
阅读全文
相关推荐
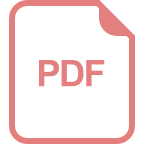
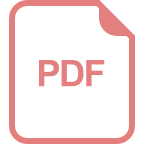
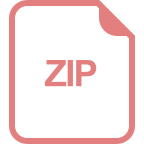











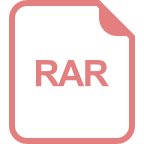

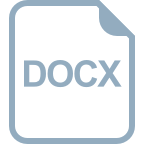