python 读取图片为数字矩阵_python读取图像矩阵文件并转换为向量实例
时间: 2024-02-20 10:58:28 浏览: 95
读取图片为数字矩阵的代码示例:
```python
from PIL import Image
# 读取图片
img = Image.open('example.jpg')
# 转换为灰度图
gray_img = img.convert('L')
# 转换为矩阵
matrix = list(gray_img.getdata())
# 打印矩阵
print(matrix)
```
将图像矩阵文件转换为向量的代码示例:
```python
import numpy as np
# 读取矩阵文件
matrix = np.loadtxt('matrix.txt')
# 将矩阵转换为向量
vector = matrix.reshape(-1)
# 打印向量
print(vector)
```
其中,`example.jpg` 是要读取的图片文件名,`matrix.txt` 是要读取的图像矩阵文件名。
相关问题
Python将一个文本文件中的多行汉字文本读取出来,用jieba对停用词处理,再向量化
要将一个文本文件中的多行汉字文本读取出来,用 jieba 对停用词处理,再向量化,可以按照以下步骤进行:
1. 使用 Python 的内置函数 open() 打开文本文件,并使用 readlines() 方法读取文件中的所有文本行,将它们存储在一个 Python 列表中:
```
with open('file.txt', 'r', encoding='utf-8') as f:
lines = f.readlines()
```
2. 导入需要使用的库,包括 jieba 库和 Scikit-learn 中的 CountVectorizer:
```
import jieba
from sklearn.feature_extraction.text import CountVectorizer
```
3. 使用 jieba 库对文本进行分词,并过滤停用词:
```
stopwords = []
with open('stopwords.txt', 'r', encoding='utf-8') as f:
for line in f:
stopwords.append(line.strip())
corpus = []
for line in lines:
words = [word for word in jieba.cut(line.strip()) if word not in stopwords]
corpus.append(' '.join(words))
```
4. 创建 CountVectorizer 的实例,并使用 fit_transform() 方法将文本行转换为向量形式:
```
vectorizer = CountVectorizer()
vectors = vectorizer.fit_transform(corpus)
```
5. 最后,可以使用 toarray() 方法将稀疏矩阵转换为 NumPy 数组:
```
array = vectors.toarray()
```
这样,文本文件中的多行汉字文本就被向量化为了一个 NumPy 数组,并且过滤了停用词。需要注意的是,这里使用的是 CountVectorizer,它将文本转换为词频矩阵。如果需要使用其他类型的向量化方法,可以参考相应的库文档进行操作。同时,需要准备好停用词表,将停用词存储在一个文本文件中。
python数据预处理实例
以下是一个简单的Python数据预处理实例,包括数据清洗、特征选择和特征缩放:
数据清洗:
```
import pandas as pd
# 读取数据
df = pd.read_csv('data.csv')
# 删除缺失值
df.dropna(inplace=True)
# 删除重复值
df.drop_duplicates(inplace=True)
```
特征选择:
```
from sklearn.feature_selection import SelectKBest
from sklearn.feature_selection import chi2
# 选择最好的K个特征
X = df.iloc[:,:-1] # 特征矩阵
y = df.iloc[:,-1] # 目标向量
selector = SelectKBest(chi2, k=5)
selector.fit(X, y)
X_new = selector.transform(X)
```
特征缩放:
```
from sklearn.preprocessing import MinMaxScaler
# 将特征缩放到0-1之间
scaler = MinMaxScaler()
X_scaled = scaler.fit_transform(X_new)
```
阅读全文
相关推荐
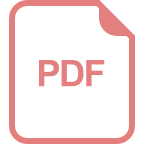
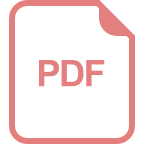
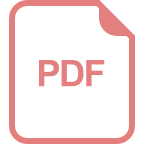
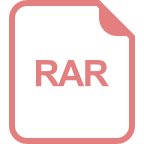
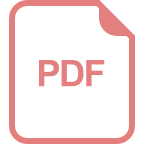
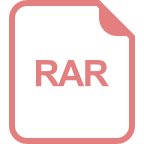
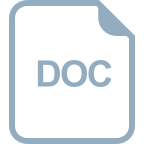
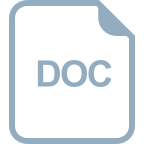
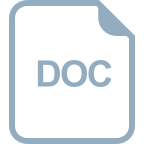
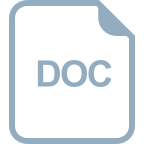
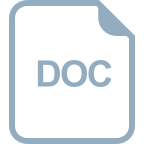
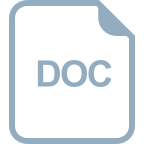
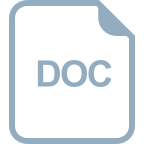
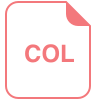
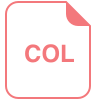