for omega in np.arange(0+dm, 3+dm, dm),在这个循环中定义omegax=omega[i]-omega[i-1],写成代码
时间: 2024-09-23 12:14:30 浏览: 35
在Python中,如果你使用NumPy库(np),并且想要在`for`循环中计算每个`omega`值与前一个`omega`之间的差,并存储在`omegax`变量中,你可以这样做:
```python
import numpy as np
# 定义步长和范围
dm = 0.1 # 假设这是你的步长
start_omega = 0 # 起始点
end_omega = 3 # 结束点
# 创建数组,包含等间距的omega值
omega = np.arange(start_omega + dm, end_omega + dm, dm)
# 初始化一个空列表来保存omegax值
omegax_list = []
# 使用for循环计算并添加到列表
for i in range(1, len(omega)):
omegax = omega[i] - omega[i-1]
omegax_list.append(omegax)
# 如果你想将结果转换为数组而不是列表,可以这样做:
omegax_array = np.array(omegax_list)
```
在这里,我们首先创建了一个从`start_omega + dm`到`end_omega + dm`的等差数列`omega`。然后,在循环中,我们计算了每个元素与前一个元素的差,并将其添加到`omegax_list`。如果你想得到一个数组形式的结果,最后一步会将这个列表转换为`numpy`数组。
相关问题
解释这行代码fig3 = plt.figure() for k in range(K): plt.semilogx(2*np.pi/fs*omega[:,k], np.arange(1,omega.shape[0]+1), linestyles[k]) fig3.suptitle('Evolution of center frequencies omega')
这行代码的作用是创建一个名为fig3的图像对象,并在其上绘制每个中心频率ω的演化曲线。具体来说,它使用for循环遍历每个中心频率,并使用plt.semilogx()函数将其表示为横坐标为2π/ fs *ω,纵坐标为1到ω.shape [0] 1的线条。linestyles [k]参数指定每个线条的样式。最后,使用fig3.suptitle()函数为整个图像添加标题:“Evolution of center frequencies omega”。
优化这段import numpy as np import matplotlib.pyplot as plt %config InlineBackend.figure_format='retina' # 输入信号 def inputVoltageSignal_func(t_vec, A, phi, noise, freq): Omega = 2np.pifreq return Anp.sin(Omegat_vec + phi) + noise * (2np.random.random(t_vec.size)-1) # 锁相测量部分 def LockinMeasurement_func(inputVoltageSignal, t_vec, ref_freq): # 生成参考信号 sin_ref = 2np.sin(2 * np.pi * ref_freq * t_vec) cos_ref = 2*np.cos(2 * np.pi * ref_freq * t_vec) # 混频信号 signal_0 = inputVoltageSignal * sin_ref signal_1 = inputVoltageSignal * cos_ref # 低通滤波 X = np.mean(signal_0) Y = np.mean(signal_1) # 计算振幅和相位 A = np.sqrt(X2 + Y2) phi = np.arctan2(Y, X) return A, phi # 参数 A = 1 phi = 0 noise = 1 ref_freq = 100 t_vec = np.linspace(0, 0.2, 1001) # 列表来保存幅值和相位数据 amplitude_list = [] phase_list = [] freq_list = np.arange(1, 1001) # 循环计算不同频率下的幅值和相位 for freq in freq_list: # 生成原始信号 Vin_vec = inputVoltageSignal_func(t_vec, A, phi, noise, freq=freq) # 锁相测量 A, phi = LockinMeasurement_func(Vin_vec, t_vec, ref_freq=freq) # 保存幅值和相位数据 amplitude_list.append(A) phase_list.append(phi) #绘图 # 幅值与频率的关系图 plt.figure(figsize=(10, 6)) plt.subplot(2,1,1) plt.plot(freq_list, amplitude_list) plt.xlabel('freq (Hz)') plt.ylabel('A') plt.title('relationship between A and freq') plt.show() # 相位与频率的关系图 plt.figure(figsize=(10, 6)) plt.subplot(2,1,2) plt.plot(freq_list, phase_list) plt.xlabel('freq (Hz)') plt.ylabel('Phi') plt.title('relationship between Phi and freq') plt.show()使用while循环
你可以将代码中的一些重复计算优化掉,并且使用一个 `while` 循环来计算不同频率下的幅值和相位。以下是优化后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
%config InlineBackend.figure_format='retina'
# 输入信号
def inputVoltageSignal_func(t_vec, A, phi, noise, freq):
Omega = 2 * np.pi * freq
return A * np.sin(Omega * t_vec + phi) + noise * (2 * np.random.random(t_vec.size) - 1)
# 锁相测量部分
def LockinMeasurement_func(inputVoltageSignal, t_vec, ref_freq):
# 生成参考信号
sin_ref = 2 * np.sin(2 * np.pi * ref_freq * t_vec)
cos_ref = 2 * np.cos(2 * np.pi * ref_freq * t_vec)
# 混频信号
signal_0 = inputVoltageSignal * sin_ref
signal_1 = inputVoltageSignal * cos_ref
# 低通滤波
X = np.mean(signal_0)
Y = np.mean(signal_1)
# 计算振幅和相位
A = np.sqrt(X**2 + Y**2)
phi = np.arctan2(Y, X)
return A, phi
# 参数
A = 1
phi = 0
noise = 1
ref_freq = 100
t_vec = np.linspace(0, 0.2, 1001)
# 列表来保存幅值和相位数据
amplitude_list = []
phase_list = []
freq_list = np.arange(1, 1001)
# 循环计算不同频率下的幅值和相位
i = 0
while i < len(freq_list):
freq = freq_list[i]
# 生成原始信号
Vin_vec = inputVoltageSignal_func(t_vec, A, phi, noise, freq=freq)
# 锁相测量
A, phi = LockinMeasurement_func(Vin_vec, t_vec, ref_freq=freq)
# 保存幅值和相位数据
amplitude_list.append(A)
phase_list.append(phi)
i += 1
# 绘图
plt.figure(figsize=(10, 6))
# 幅值与频率的关系图
plt.subplot(2, 1, 1)
plt.plot(freq_list, amplitude_list)
plt.xlabel('freq (Hz)')
plt.ylabel('A')
plt.title('relationship between A and freq')
# 相位与频率的关系图
plt.subplot(2, 1, 2)
plt.plot(freq_list, phase_list)
plt.xlabel('freq (Hz)')
plt.ylabel('Phi')
plt.title('relationship between Phi and freq')
plt.tight_layout()
plt.show()
```
这样,你可以使用 `while` 循环来计算不同频率下的幅值和相位,并且一次性绘制出幅值与频率的关系图和相位与频率的关系图。
阅读全文
相关推荐
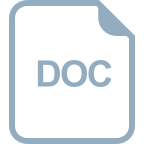
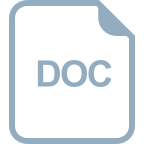
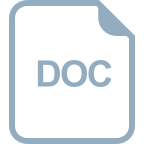











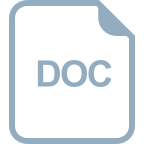
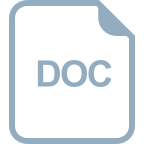
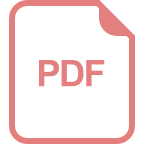