esp32 arduino 下载http服务器的bin文件到SD卡中的代码
时间: 2024-09-10 12:20:24 浏览: 134
要在ESP32上使用Arduino IDE下载HTTP服务器的bin文件到SD卡中,你需要进行几个步骤。首先,确保你的Arduino IDE环境已经安装了ESP32的支持库,并且你有一个SD卡模块与ESP32连接。以下是一个简单的示例代码,展示了如何通过HTTP服务器下载bin文件并将其保存到SD卡中。
```cpp
#include <WiFi.h>
#include <HTTPClient.h>
#include <FS.h>
#include <SD.h>
const char* ssid = "你的WiFi名称";
const char* password = "你的WiFi密码";
const char* serverName = "服务器的域名或IP";
const int httpPort = 80;
void setup() {
Serial.begin(115200);
// 初始化SD卡
if (!SD.begin()) {
Serial.println("SD卡初始化失败!");
return;
}
// 连接WiFi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("已连接到WiFi网络,IP地址:");
Serial.println(WiFi.localIP());
// 打开文件以便写入
File file = SD.open("/server.bin", FILE_WRITE);
if (!file) {
Serial.println("无法打开文件");
return;
}
}
void loop() {
if(WiFi.status() == WL_CONNECTED) {
HTTPClient http;
String url = String("http://") + serverName + "/path/to/your/binfile.bin";
http.begin(url);
int httpCode = http.GET();
if (httpCode > 0) {
// 服务器响应状态码
Serial.print("状态码:");
Serial.println(httpCode);
if (httpCode == HTTP_CODE_OK) {
// 读取数据并写入SD卡
Stream& response = http.getStream();
while (response.available()) {
file.write(response.read());
}
Serial.println("文件下载成功!");
}
} else {
Serial.print("下载失败,HTTP错误:");
Serial.println(httpCode);
}
http.end();
} else {
Serial.println("WiFi断开连接");
}
delay(10000); // 等待10秒后再次尝试
}
```
这段代码首先通过WiFi连接到你的网络,然后尝试从指定的URL下载bin文件,之后将文件内容写入SD卡中。请注意,你需要替换`ssid`, `password`, `serverName`以及文件的`url`路径为你实际的网络信息和服务器文件路径。
阅读全文
相关推荐
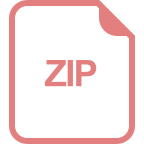
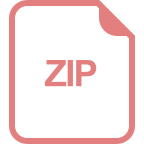















