使用C语言写一段使用pthread_create函数的代码
时间: 2023-05-12 11:04:31 浏览: 104
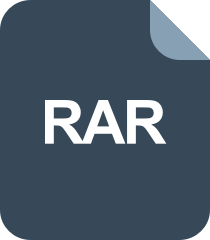
Program using Pthreads

好的,以下是一段使用pthread_create函数的C语言代码:
```
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
printf("Hello from thread!\n");
return NULL;
}
int main() {
pthread_t thread_id;
int ret = pthread_create(&thread_id, NULL, thread_func, NULL);
if (ret != 0) {
printf("Error creating thread!\n");
return 1;
}
printf("Hello from main thread!\n");
pthread_join(thread_id, NULL);
return 0;
}
```
这段代码创建了一个新的线程,该线程调用thread_func函数并打印一条消息。主线程也打印了一条消息,然后等待新线程结束。
阅读全文
相关推荐
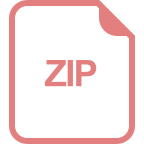
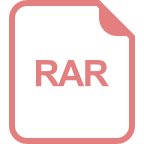















