stm32矩阵键盘4x4
时间: 2023-06-30 21:14:20 浏览: 111
STM32 矩阵键盘 4x4 是一种常见的输入设备,可以通过 STM32 的 GPIO 以矩阵的形式将其连接到 MCU 上。下面是连接步骤:
1. 将矩阵键盘的行引脚连接到 STM32 的输出引脚,列引脚连接到 STM32 的输入引脚。
2. 设置 STM32 的输出引脚为推挽输出模式,输入引脚为上拉输入模式。
3. 定义一个 4x4 的矩阵数组,记录每个按键的状态。
4. 在主循环中,轮询每个列引脚的状态,如果有按键按下,则将对应的行和列的值更新到矩阵数组中。
下面是一个简单的示例代码:
```c
#include "stm32f10x.h"
#define ROW_NUM 4
#define COL_NUM 4
uint8_t key_matrix[ROW_NUM][COL_NUM] = {
{1, 2, 3, 'A'},
{4, 5, 6, 'B'},
{7, 8, 9, 'C'},
{'*', 0, '#', 'D'}
};
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void keypad_scan(void)
{
int i, j;
uint8_t col_state;
for (i = 0; i < COL_NUM; i++) {
GPIO_SetBits(GPIOB, GPIO_Pin_4 + i); // 激活列
col_state = GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_0) |
GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_1) << 1 |
GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_2) << 2 |
GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_3) << 3;
for (j = 0; j < ROW_NUM; j++) {
if (col_state == (1 << j)) {
// 检测到按键按下,更新矩阵数组
key_matrix[j][i] = 1;
}
}
GPIO_ResetBits(GPIOB, GPIO_Pin_4 + i); // 关闭列
}
}
int main(void)
{
GPIO_Configuration();
while (1) {
keypad_scan();
}
}
```
这是一个简单的矩阵键盘扫描程序,你可以根据自己的需要进行修改。
阅读全文
相关推荐

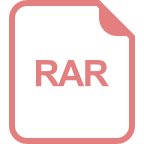


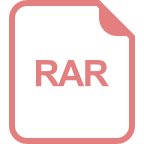
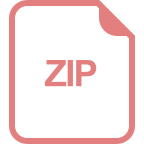
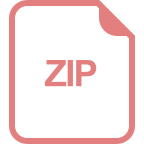
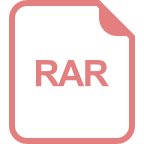
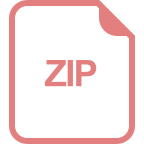
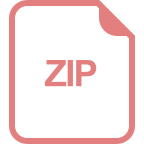
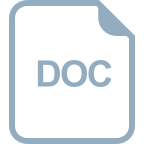
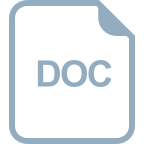



