写一个识别茶杯的模型代码
时间: 2024-02-06 10:08:59 浏览: 78
首先,需要使用深度学习框架(例如TensorFlow或PyTorch)来建立模型。下面是一个使用TensorFlow的茶杯识别模型的示例代码:
```
import tensorflow as tf
# 定义模型
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(224, 224, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Conv2D(128, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(256, activation='relu'),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# 编译模型
model.compile(loss='binary_crossentropy',
optimizer=tf.keras.optimizers.RMSprop(lr=0.001),
metrics=['accuracy'])
# 训练模型
train_datagen = tf.keras.preprocessing.image.ImageDataGenerator(rescale=1./255)
train_generator = train_datagen.flow_from_directory(
'tea_cup_dataset/train',
target_size=(224, 224),
batch_size=20,
class_mode='binary')
validation_datagen = tf.keras.preprocessing.image.ImageDataGenerator(rescale=1./255)
validation_generator = validation_datagen.flow_from_directory(
'tea_cup_dataset/validation',
target_size=(224, 224),
batch_size=20,
class_mode='binary')
history = model.fit(
train_generator,
steps_per_epoch=100,
epochs=10,
validation_data=validation_generator,
validation_steps=50,
verbose=2)
# 使用模型进行预测
test_datagen = tf.keras.preprocessing.image.ImageDataGenerator(rescale=1./255)
test_generator = test_datagen.flow_from_directory(
'tea_cup_dataset/test',
target_size=(224, 224),
batch_size=20,
class_mode='binary')
test_loss, test_acc = model.evaluate(test_generator, steps=50)
print('Test accuracy:', test_acc)
```
上述代码中,我们使用了卷积神经网络(Convolutional Neural Network)来构建模型。该模型包含了三个卷积层和两个全连接层,其中每个卷积层后面都跟着一个最大池化层。最后一个输出层使用sigmoid函数作为激活函数,用于判断输入图像是否为茶杯。
在训练模型时,我们使用ImageDataGenerator从文件夹中读取图像数据,并将它们预处理为模型所需的格式。我们还指定了训练集、验证集和测试集的目录,并设置了每个批次的大小和迭代次数。
最后,我们使用evaluate方法对模型进行评估,并输出测试集的准确率。
阅读全文
相关推荐
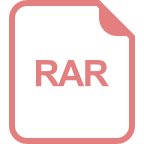
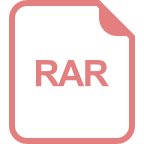
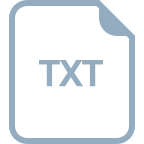
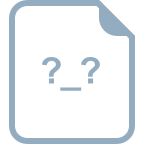
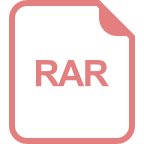
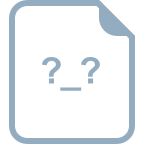
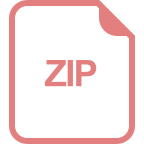
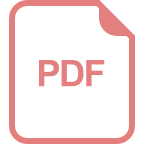
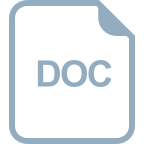
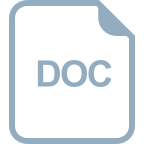
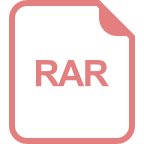
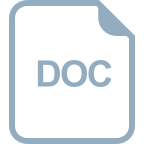
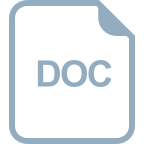

