用C语言且使用链表头插法写一个贪吃蛇的代码
时间: 2024-01-06 11:06:32 浏览: 89
好的,以下是使用C语言并使用链表头插法实现的简单贪吃蛇代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#define WIDTH 40
#define HEIGHT 20
enum DIRECTION { STOP = 0, LEFT, RIGHT, UP, DOWN };
typedef struct Node {
int x;
int y;
struct Node* next;
} Node;
Node* head = NULL;
Node* tail = NULL;
int fruitX, fruitY;
enum DIRECTION dir;
int score;
int gameover;
void init_snake() {
head = (Node*)malloc(sizeof(Node));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
tail = head;
dir = STOP;
score = 0;
gameover = 0;
}
void print_board() {
system("cls");
printf("Score: %d\n", score);
for (int i = 0; i <= WIDTH + 1; i++) printf("#");
printf("\n");
for (int i = 0; i < HEIGHT; i++) {
printf("#");
for (int j = 0; j < WIDTH; j++) {
if (j == head->x && i == head->y) printf("O");
else if (j == fruitX && i == fruitY) printf("F");
else {
Node* p = head->next;
int printed = 0;
while (p) {
if (j == p->x && i == p->y) {
printf("o");
printed = 1;
break;
}
p = p->next;
}
if (!printed) printf(" ");
}
}
printf("#\n");
}
for (int i = 0; i <= WIDTH + 1; i++) printf("#");
printf("\n");
}
void generate_fruit() {
srand(time(NULL));
fruitX = rand() % WIDTH;
fruitY = rand() % HEIGHT;
}
void add_node(int x, int y) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->x = x;
new_node->y = y;
new_node->next = head;
head = new_node;
}
void remove_tail() {
Node* p = head;
while (p->next != tail) p = p->next;
p->next = NULL;
free(tail);
tail = p;
}
void input() {
if (_kbhit()) {
switch (_getch()) {
case 'a':
if (dir != RIGHT) dir = LEFT;
break;
case 'd':
if (dir != LEFT) dir = RIGHT;
break;
case 'w':
if (dir != DOWN) dir = UP;
break;
case 's':
if (dir != UP) dir = DOWN;
break;
case 'x':
gameover = 1;
break;
}
}
}
void logic() {
// update snake position
switch (dir) {
case LEFT:
add_node(head->x - 1, head->y);
break;
case RIGHT:
add_node(head->x + 1, head->y);
break;
case UP:
add_node(head->x, head->y - 1);
break;
case DOWN:
add_node(head->x, head->y + 1);
break;
}
// check if snake hits the wall or itself
if (head->x < 0 || head->x >= WIDTH || head->y < 0 || head->y >= HEIGHT) {
gameover = 1;
return;
}
Node* p = head->next;
while (p) {
if (head->x == p->x && head->y == p->y) {
gameover = 1;
return;
}
p = p->next;
}
// check if snake eats the fruit
if (head->x == fruitX && head->y == fruitY) {
generate_fruit();
score++;
}
else {
remove_tail();
}
}
int main() {
init_snake();
generate_fruit();
while (!gameover) {
print_board();
input();
logic();
}
printf("Game Over! Your final score is %d.\n", score);
return 0;
}
```
这个代码示例使用了链表头插法来维护贪吃蛇的身体,实现了基本的游戏逻辑。然而,这个代码示例还有很多可以改进和优化的地方,比如添加更多的游戏元素和难度级别,使用更复杂的算法来控制贪吃蛇的移动等等。
阅读全文
相关推荐
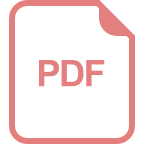
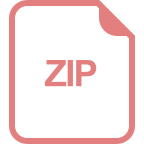
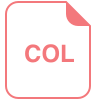

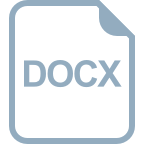
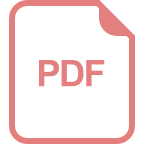
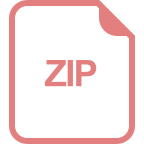
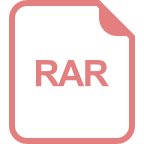
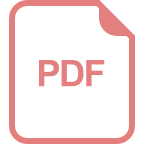
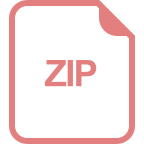