时间片轮转算法实现进程创建按钮,并用表格动态显示调度过程的javaGUI实现
时间: 2023-06-14 19:04:21 浏览: 93
以下是一个简单的时间片轮转调度算法的 Java 代码,包括进程创建按钮和表格动态显示调度过程的 GUI 实现。该代码仅供参考。
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.LinkedList;
public class TimeSliceScheduler extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private static final int TIME_SLICE = 2; // 时间片大小,单位为秒
private JLabel titleLabel, nameLabel, timeLabel;
private JTextField nameField, timeField;
private JButton createButton, startButton;
private JTable table;
private DefaultTableModel model;
private Timer timer;
private LinkedList<Process> queue; // 进程队列
private Process currentProcess; // 当前正在执行的进程
private int currentTime; // 当前时间
public TimeSliceScheduler() {
super("时间片轮转调度算法");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 400);
setLocationRelativeTo(null);
setLayout(null);
// 标题
titleLabel = new JLabel("时间片轮转调度算法");
titleLabel.setFont(new Font("宋体", Font.BOLD, 20));
titleLabel.setBounds(150, 20, 200, 30);
add(titleLabel);
// 进程名输入框和标签
nameLabel = new JLabel("进程名:");
nameLabel.setBounds(50, 70, 60, 30);
add(nameLabel);
nameField = new JTextField();
nameField.setBounds(110, 70, 100, 30);
add(nameField);
// 执行时间输入框和标签
timeLabel = new JLabel("执行时间:");
timeLabel.setBounds(230, 70, 60, 30);
add(timeLabel);
timeField = new JTextField();
timeField.setBounds(290, 70, 100, 30);
add(timeField);
// 创建进程按钮
createButton = new JButton("创建进程");
createButton.setBounds(410, 70, 80, 30);
createButton.addActionListener(this);
add(createButton);
// 进程表格
String[] columnNames = {"进程名", "到达时间", "执行时间", "开始时间", "完成时间", "周转时间", "带权周转时间"};
model = new DefaultTableModel(columnNames, 0);
table = new JTable(model);
JScrollPane scrollPane = new JScrollPane(table);
scrollPane.setBounds(50, 120, 440, 180);
add(scrollPane);
// 开始按钮
startButton = new JButton("开始调度");
startButton.setBounds(200, 320, 100, 30);
startButton.addActionListener(this);
add(startButton);
setVisible(true);
}
public static void main(String[] args) {
new TimeSliceScheduler();
}
// 处理按钮点击事件
public void actionPerformed(ActionEvent e) {
if (e.getSource() == createButton) { // 创建进程按钮
String name = nameField.getText().trim();
int time = Integer.parseInt(timeField.getText().trim());
Process process = new Process(name, time);
queue.add(process);
Object[] rowData = {process.getName(), process.getArrivalTime(), process.getExecutionTime(), "", "", "", ""};
model.addRow(rowData);
nameField.setText("");
timeField.setText("");
} else if (e.getSource() == startButton) { // 开始调度按钮
startButton.setEnabled(false);
timer.start();
}
}
// 时间片轮转调度算法
public void schedule() {
if (currentProcess == null) { // 如果当前没有进程在执行,则从队列中取出第一个进程执行
if (queue.isEmpty()) { // 如果队列为空,则调度结束
timer.stop();
return;
}
currentProcess = queue.removeFirst();
currentProcess.setStartTime(currentTime);
}
currentProcess.setExecutionTime(currentProcess.getExecutionTime() - TIME_SLICE); // 执行时间减少
if (currentProcess.getExecutionTime() <= 0) { // 如果进程已经执行完毕,则更新进程信息并执行下一个进程
currentProcess.setFinishTime(currentTime + TIME_SLICE);
currentProcess.calcTurnaroundTime();
currentProcess.calcWeightedTurnaroundTime();
int row = table.getSelectedRow();
model.setValueAt(currentProcess.getStartTime(), row, 3);
model.setValueAt(currentProcess.getFinishTime(), row, 4);
model.setValueAt(currentProcess.getTurnaroundTime(), row, 5);
model.setValueAt(currentProcess.getWeightedTurnaroundTime(), row, 6);
currentProcess = null;
} else { // 如果进程还未执行完毕,则继续执行该进程
queue.addLast(currentProcess);
currentProcess = null;
}
currentTime += TIME_SLICE;
}
// 进程类
class Process {
private String name; // 进程名
private int arrivalTime; // 到达时间
private int executionTime; // 执行时间
private int startTime; // 开始时间
private int finishTime; // 完成时间
private int turnaroundTime; // 周转时间
private double weightedTurnaroundTime; // 带权周转时间
public Process(String name, int executionTime) {
this.name = name;
this.executionTime = executionTime;
this.arrivalTime = currentTime;
}
public String getName() {
return name;
}
public int getArrivalTime() {
return arrivalTime;
}
public int getExecutionTime() {
return executionTime;
}
public void setExecutionTime(int executionTime) {
this.executionTime = executionTime;
}
public int getStartTime() {
return startTime;
}
public void setStartTime(int startTime) {
this.startTime = startTime;
}
public int getFinishTime() {
return finishTime;
}
public void setFinishTime(int finishTime) {
this.finishTime = finishTime;
}
public int getTurnaroundTime() {
return turnaroundTime;
}
public void calcTurnaroundTime() {
this.turnaroundTime = finishTime - arrivalTime;
}
public double getWeightedTurnaroundTime() {
return weightedTurnaroundTime;
}
public void calcWeightedTurnaroundTime() {
this.weightedTurnaroundTime = turnaroundTime * 1.0 / executionTime;
}
}
}
```
运行该程序,将会出现一个窗口,可以通过创建进程按钮来创建进程,然后点击开始调度按钮来开始时间片轮转调度算法。在表格中可以动态显示进程的调度过程,包括进程的开始时间、完成时间、周转时间和带权周转时间等信息。
相关推荐
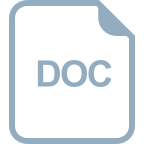










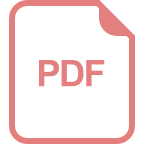