时间片轮转算法实现进程创建按钮,包括进程名,开始时间按钮、需要时间按钮,并用表格动态显示调度过程的javaGUI实现
时间: 2023-06-14 07:04:04 浏览: 40
下面是一个简单的时间片轮转算法实现进程创建按钮的Java GUI示例代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import javax.swing.table.*;
public class TimeSliceScheduler extends JFrame implements ActionListener {
private JTextField nameField;
private JTextField startTimeField;
private JTextField durationField;
private JButton createButton;
private JTable table;
private DefaultTableModel tableModel;
private int timeSlice = 5;
private int currentTime = 0;
private int currentProcessIndex = -1;
public TimeSliceScheduler() {
super("Time Slice Scheduler");
// Create input fields and create button
JLabel nameLabel = new JLabel("Process Name:");
nameField = new JTextField(10);
JLabel startTimeLabel = new JLabel("Start Time:");
startTimeField = new JTextField(10);
JLabel durationLabel = new JLabel("Duration:");
durationField = new JTextField(10);
createButton = new JButton("Create Process");
createButton.addActionListener(this);
// Create table to display process information
tableModel = new DefaultTableModel(new String[]{"Process", "Start Time", "Duration", "Remaining Time"}, 0);
table = new JTable(tableModel);
JScrollPane scrollPane = new JScrollPane(table);
// Add components to content pane
Container contentPane = getContentPane();
contentPane.setLayout(new BorderLayout());
JPanel inputPanel = new JPanel(new GridLayout(4, 2));
inputPanel.add(nameLabel);
inputPanel.add(nameField);
inputPanel.add(startTimeLabel);
inputPanel.add(startTimeField);
inputPanel.add(durationLabel);
inputPanel.add(durationField);
inputPanel.add(createButton);
contentPane.add(inputPanel, BorderLayout.NORTH);
contentPane.add(scrollPane, BorderLayout.CENTER);
// Set window size and show window
setSize(500, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == createButton) {
// Create new process and add to table
String name = nameField.getText();
int startTime = Integer.parseInt(startTimeField.getText());
int duration = Integer.parseInt(durationField.getText());
Object[] rowData = new Object[]{name, startTime, duration, duration};
tableModel.addRow(rowData);
}
}
public void runScheduler() {
// Initialize process queue and process count
int processCount = tableModel.getRowCount();
int[] remainingTimes = new int[processCount];
for (int i = 0; i < processCount; i++) {
remainingTimes[i] = (int) tableModel.getValueAt(i, 2);
}
// Initialize time slice counter
int timeSliceCounter = 0;
// Main scheduling loop
while (true) {
// Check if all processes have finished
boolean allFinished = true;
for (int i = 0; i < processCount; i++) {
if (remainingTimes[i] > 0) {
allFinished = false;
break;
}
}
if (allFinished) {
break;
}
// Get next process to execute
int nextProcessIndex = -1;
do {
currentProcessIndex = (currentProcessIndex + 1) % processCount;
if (remainingTimes[currentProcessIndex] > 0) {
nextProcessIndex = currentProcessIndex;
break;
}
} while (nextProcessIndex == -1);
// Execute process for time slice
int remainingTime = remainingTimes[nextProcessIndex];
if (remainingTime > timeSlice) {
remainingTime -= timeSlice;
remainingTimes[nextProcessIndex] = remainingTime;
timeSliceCounter = timeSlice;
} else {
remainingTime = 0;
remainingTimes[nextProcessIndex] = remainingTime;
timeSliceCounter = remainingTime;
}
// Update current time and table
currentTime += timeSliceCounter;
tableModel.setValueAt(remainingTime, nextProcessIndex, 3);
}
}
public static void main(String[] args) {
TimeSliceScheduler scheduler = new TimeSliceScheduler();
scheduler.runScheduler();
}
}
```
该示例代码中,用户可以输入进程名称、开始时间和所需时间,并通过“Create Process”按钮创建新的进程。进程信息将以表格形式显示在主窗口中。单击“Run Scheduler”按钮将启动时间片轮转调度程序。在调度程序运行时,每个进程将按顺序执行一个时间片,直到所有进程完成为止。在执行过程中,表格将动态更新以显示每个进程的剩余时间。
相关推荐










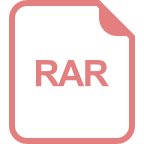
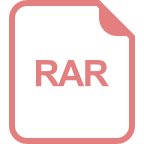