使用java写一个微信机器人的程序
时间: 2024-06-08 17:05:11 浏览: 19
要使用Java编写一个微信机器人程序,你需要先了解微信开放平台的接口文档和Java开发环境的相关知识。
以下是一个简单的微信机器人程序示例,它可以自动回复用户发送的文本消息:
```java
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
public class WeChatRobot {
private static final String API_URL = "https://api.weixin.qq.com/cgi-bin/";
private String accessToken;
public WeChatRobot(String appId, String appSecret) {
accessToken = getAccessToken(appId, appSecret);
}
private String getAccessToken(String appId, String appSecret) {
String url = API_URL + "token?grant_type=client_credential&appid=" + appId + "&secret=" + appSecret;
String result = sendGet(url);
JSONObject json = JSON.parseObject(result);
return json.getString("access_token");
}
public void handleMessage(JSONObject message) {
String fromUser = message.getString("FromUserName");
String toUser = message.getString("ToUserName");
String msgType = message.getString("MsgType");
String content = message.getString("Content");
if ("text".equals(msgType)) {
String reply = "你发送的消息是:" + content;
sendMessage(fromUser, toUser, reply);
}
}
private void sendMessage(String fromUser, String toUser, String content) {
String url = API_URL + "message/custom/send?access_token=" + accessToken;
Map<String, Object> message = new HashMap<>();
message.put("touser", fromUser);
message.put("msgtype", "text");
Map<String, String> text = new HashMap<>();
text.put("content", content);
message.put("text", text);
String json = JSON.toJSONString(message);
sendPost(url, json);
}
private String sendGet(String url) {
try {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
InputStream is = con.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(is));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
private String sendPost(String url, String json) {
try {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setDoOutput(true);
con.getOutputStream().write(json.getBytes());
InputStream is = con.getInputStream();
BufferedReader in = new BufferedReader(new InputStreamReader(is));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
return response.toString();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
public static void main(String[] args) {
String appId = "你的AppID";
String appSecret = "你的AppSecret";
WeChatRobot robot = new WeChatRobot(appId, appSecret);
while (true) {
String url = API_URL + "message/custom/send?access_token=" + robot.accessToken;
String result = robot.sendGet(url);
JSONObject json = JSON.parseObject(result);
int errcode = json.getIntValue("errcode");
if (errcode == 0) {
JSONObject message = json.getJSONObject("message");
robot.handleMessage(message);
} else {
System.out.println("获取消息失败,错误码:" + errcode);
}
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
在这个示例中,我们使用了阿里巴巴的 fastjson 库来处理 JSON 数据,使用 HttpURLConnection 类来发送 HTTP 请求。在主函数中,我们使用一个死循环来不断地获取用户发来的消息,并调用 handleMessage 方法来处理消息。当收到文本消息时,我们会回复用户发送的文本内容。
在实际的开发中,你需要根据微信开放平台的接口文档,选择合适的接口来实现你的微信机器人功能。同时,你需要注意保护用户的隐私信息,并遵循微信开放平台的规定和政策。
相关推荐
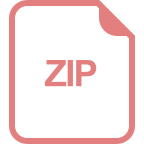
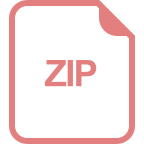
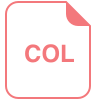
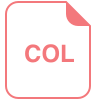
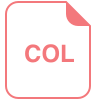
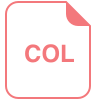
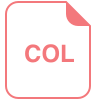









