请用c语言编写求二叉树深度的递归算法
时间: 2024-11-16 16:20:35 浏览: 27
在C语言中,可以使用递归来计算二叉树的深度。这里是一个简单的递归函数示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
} TreeNode;
// 计算二叉树深度的辅助函数
int maxDepth(struct TreeNode* root) {
// 如果根节点为空,返回0
if (root == NULL) return 0;
// 否则分别计算左子树和右子树的深度,并取较大值加1作为当前的高度
int leftDepth = maxDepth(root->left);
int rightDepth = maxDepth(root->right);
// 返回最大深度
return (leftDepth > rightDepth ? leftDepth : rightDepth) + 1;
}
int main() {
// 创建一个简单的二叉树实例并测试这个函数
TreeNode* root = (TreeNode*)malloc(sizeof(TreeNode));
root->val = 1;
root->left = (TreeNode*)malloc(sizeof(TreeNode));
root->left->val = 2;
root->right = (TreeNode*)malloc(sizeof(TreeNode));
root->right->val = 3;
int depth = maxDepth(root);
printf("The maximum depth of the binary tree is %d\n", depth);
// 清理内存
free(root->left);
free(root->right);
free(root);
return 0;
}
```
在这个例子中,`maxDepth` 函数会一直递归地检查左右子树,直到找到空节点,然后返回当前层数。当处理完整个树后,最终的深度即为树的最大深度。
阅读全文
相关推荐
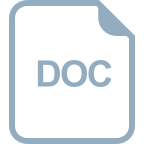
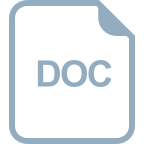
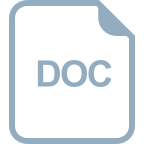



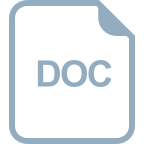











