c++ 重载运算符“-”,实现复数的共轭复数
时间: 2023-08-18 08:07:48 浏览: 203
要实现复数的共轭复数运算,可以重载运算符“-”,然后在函数中返回一个新的复数对象,该对象的实部与原对象相同,虚部的符号取反。以下是示例代码:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag; // 复数的实部和虚部
public:
Complex(double r = 0, double i = 0): real(r), imag(i) {} // 构造函数
Complex operator-() const { // 重载运算符“-”
return Complex(real, -imag); // 返回共轭复数
}
void display() const { // 输出复数
cout << "(" << real << ", " << imag << "i)" << endl;
}
};
int main() {
Complex c1(3, 4), c2;
c2 = -c1; // 调用重载的“-”运算符
c1.display(); // 输出原复数
c2.display(); // 输出共轭复数
return 0;
}
```
输出结果为:
```
(3, 4i)
(3, -4i)
```
在示例代码中,通过重载运算符“-”返回共轭复数对象,然后在主函数中调用该运算符实现求解。
相关问题
c++重载运算符==复数表示
在 C++ 中,可以通过重载运算符来定义复数的相等性比较。下面是一个示例实现:
```c++
#include <iostream>
using namespace std;
class Complex {
private:
double real;
double imag;
public:
Complex(double r = 0.0, double i = 0.0) {
real = r;
imag = i;
}
bool operator==(const Complex& other) const {
return (real == other.real) && (imag == other.imag);
}
};
int main() {
Complex a(3.0, 4.0);
Complex b(3.0, 4.0);
Complex c(5.0, 6.0);
cout << boolalpha;
cout << (a == b) << endl; // 输出 true
cout << (a == c) << endl; // 输出 false
return 0;
}
```
在上面的示例中,我们重载了 `==` 运算符,实现了复数之间的相等性比较。注意,我们使用了常量引用作为参数,并且在函数体内使用了 `const` 修饰符,以确保不会修改被比较的对象。
为复数类增加重载运算符-、-=用友元函数
可以这样实现:
```c++
#include <iostream>
class Complex {
public:
Complex(double r = 0, double i = 0): real(r), imag(i) {}
// 定义友元函数
friend Complex operator-(const Complex& c1, const Complex& c2);
friend Complex operator-=(Complex& c1, const Complex& c2);
void display() const {
std::cout << real << " + " << imag << "i" << std::endl;
}
private:
double real;
double imag;
};
// 重载运算符-,返回一个新的复数类
Complex operator-(const Complex& c1, const Complex& c2) {
return Complex(c1.real - c2.real, c1.imag - c2.imag);
}
// 重载运算符-=,修改原有的复数类
Complex operator-=(Complex& c1, const Complex& c2) {
c1.real -= c2.real;
c1.imag -= c2.imag;
return c1;
}
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 - c2;
std::cout << "c3 = ";
c3.display(); // 输出:c3 = -2 + -2i
c1 -= c2;
std::cout << "c1 = ";
c1.display(); // 输出:c1 = -2 + -2i
return 0;
}
```
在上面的代码中,我们为 `Complex` 类增加了两个友元函数 `operator-` 和 `operator-=`,分别用于实现重载运算符 `-` 和 `-=`。`operator-` 重载运算符 `-`,返回一个新的 `Complex` 类,而 `operator-=` 重载运算符 `-=`,修改原有的 `Complex` 类。注意,在 `operator-=` 中,我们返回的是修改后的 `c1`,这样可以支持链式操作。
阅读全文
相关推荐
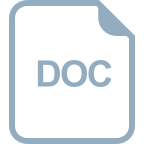
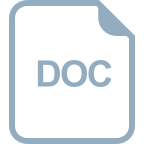
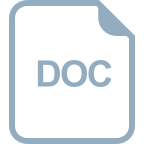
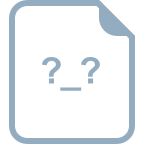
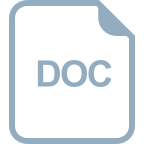
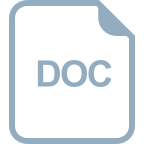
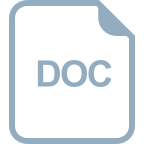






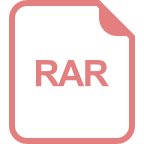

