PLSA主题模型代码
时间: 2023-02-08 08:36:58 浏览: 192
PLSA(Probabilistic Latent Semantic Analysis)主题模型的代码实现可以使用 Python 来编写。
以下是一个简单的 PLSA 代码示例(来自 Python 的 scikit-learn 库):
```python
from sklearn.decomposition import LatentDirichletAllocation
# 构造词袋数据
data = # 词袋数据
# 初始化模型
lda = LatentDirichletAllocation(n_components=10)
# 训练模型
lda.fit(data)
# 查看模型主题
print(lda.components_)
```
其中,n_components 参数表示要建立的主题个数,fit 函数用于训练模型,components_ 属性存储着每个主题中各个单词的权重。
需要注意的是,本示例中的 LDA 模型本质上就是 PLSA 的一种变形,就是已经确定了各维度之间的关系和分布。
如果希望了解更详细的实现细节和更多参数配置,请参考 scikit-learn 官方文档。
相关问题
R语言下plsa主题建模的代码
以下是在R语言下使用plsa主题建模的代码示例:
```R
# 载入plsa包
library(pls)
# 读取文本数据
mydata <- readLines("mydata.txt")
# 将文本数据转化为文档-词频矩阵
mycorpus <- Corpus(VectorSource(mydata))
tdm <- TermDocumentMatrix(mycorpus)
# 定义主题数
K <- 10
# 建立plsa模型
myplsa <- pls(tdm, K)
# 查看主题-词分布矩阵
myplsa$topic.word
# 查看文档-主题分布矩阵
myplsa$document.topic
```
在此代码示例中,首先使用`plsa`包读取文本数据,并将其转化为文档-词频矩阵。然后,定义主题数`K`,并使用`pls`函数建立plsa模型。最后,通过查看主题-词分布矩阵和文档-主题分布矩阵,可以得到主题模型的结果。需要注意的是,plsa模型的结果需要进行解释和分析,以便对文本数据进行进一步的理解和应用。
对pLSA、LDA,DMM模型调参,使用困惑度指标选择最近K,计算困惑度,给出python代码
好的,以下是使用困惑度指标选择最优参数的 Python 代码示例:
首先,我们需要导入相应的库:
```python
import numpy as np
from sklearn.datasets import fetch_20newsgroups
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.decomposition import NMF, LatentDirichletAllocation
from gensim.models import LdaModel
from gensim.corpora import Dictionary
from gensim.test.utils import common_corpus, common_dictionary
from gensim.models.wrappers import DtmModel
from gensim.models.wrappers.dtmmodel import DtmParameters
from sklearn.model_selection import train_test_split
```
然后,我们可以使用 `fetch_20newsgroups` 函数加载 20 Newsgroups 数据集,并将其划分为训练集和测试集:
```python
data = fetch_20newsgroups(subset='all', shuffle=True, random_state=42)
X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.2, random_state=42)
```
接着,我们需要将文本数据转换成词袋向量,以便用于模型训练:
```python
vectorizer = CountVectorizer(max_features=5000, stop_words='english')
X_train_vec = vectorizer.fit_transform(X_train)
X_test_vec = vectorizer.transform(X_test)
```
然后,我们可以定义一个函数来训练不同的主题模型,并计算在测试集上的困惑度:
```python
def train_model(model_type, k_range):
perplexity = []
for k in k_range:
if model_type == 'pLSA':
model = NMF(n_components=k, init='nndsvd', random_state=42)
model.fit(X_train_vec)
perplexity.append(model.reconstruction_err_)
elif model_type == 'LDA':
model = LatentDirichletAllocation(n_components=k, learning_method='online', random_state=42)
model.fit(X_train_vec)
perplexity.append(model.perplexity(X_test_vec))
elif model_type == 'DMM':
dictionary = Dictionary(common_corpus)
corpus = [dictionary.doc2bow(text) for text in X_train]
params = DtmParameters(alpha=0.01, num_topics=k, id2word=dictionary, time_slices=[len(X_train)], initialize_lda=True)
model = DtmModel('/path/to/dtm/binary', corpus=corpus, time_slices=[len(X_train)], id2word=dictionary, num_topics=k, params=params)
perplexity.append(model.log_perplexity(corpus))
return perplexity
```
在这个函数中,我们首先定义了一个列表 `perplexity`,用于存储在不同主题数下的困惑度。然后,根据传入的模型类型和主题数范围,分别训练不同的主题模型,并计算在测试集上的困惑度。最后,返回困惑度列表。
接下来,我们可以调用 `train_model` 函数来训练不同的模型,并选择困惑度最小的模型作为最优模型:
```python
k_range = range(5, 50, 5)
perplexity_plsa = train_model('pLSA', k_range)
perplexity_lda = train_model('LDA', k_range)
perplexity_dmm = train_model('DMM', k_range)
min_perplexity_plsa = np.argmin(perplexity_plsa)
min_perplexity_lda = np.argmin(perplexity_lda)
min_perplexity_dmm = np.argmin(perplexity_dmm)
print('The optimal number of topics for pLSA is', k_range[min_perplexity_plsa])
print('The optimal number of topics for LDA is', k_range[min_perplexity_lda])
print('The optimal number of topics for DMM is', k_range[min_perplexity_dmm])
```
在这个示例中,我们尝试了 5 到 50 个主题数,每隔 5 个尝试一次。然后,分别计算了 pLSA、LDA 和 DMM 模型在不同主题数下的困惑度,并选择困惑度最小的模型作为最优模型。最后,打印出最优的主题数。
希望这个示例能够帮到你!
阅读全文
相关推荐
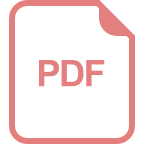
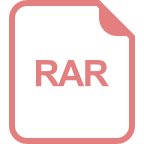
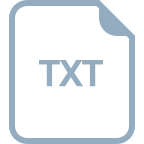
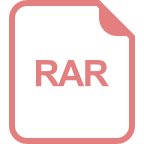
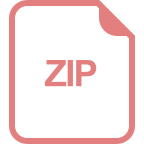
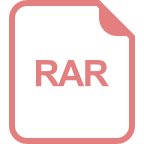
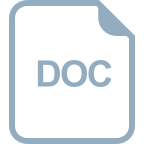
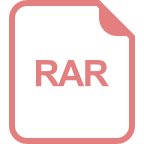
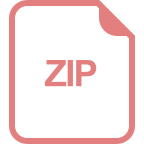
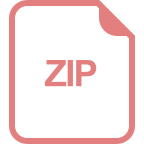
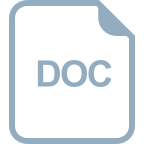
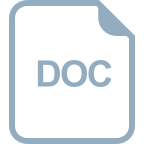
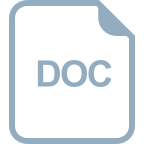
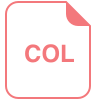

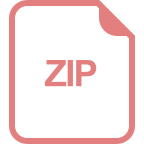