用C++采用改进算法(时间复杂性为O(n2)),实现求最大子段和,并统计运行时间。
时间: 2024-12-10 14:47:04 浏览: 14
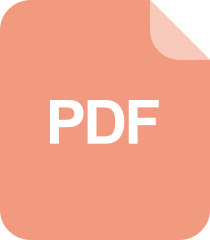
2023 CSP-J1 CSP-S1 初赛 第1轮 各种排序算法的比较.pdf
在C++中,可以使用Kadane's Algorithm (也称为动态规划算法) 来求解最大子段和的问题,其时间复杂度为O(n),而非O(n^2)。Kadane's Algorithm的基本思想是不断更新当前已知的最大子数组和,同时保持对全局最大子数组和的记录。
以下是使用Kadane's Algorithm的C++代码示例:
```cpp
#include <iostream>
#include <climits> // 包含INT_MAX用于处理负数情况
int maxSubarraySum(int arr[], int size) {
int curr_max = arr[0]; // 当前最大子数组和
int global_max = arr[0]; // 全局最大子数组和
for (int i = 1; i < size; i++) {
curr_max = std::max(arr[i], curr_max + arr[i]); // 更新当前最大值
if (curr_max > global_max)
global_max = curr_max; // 如果当前最大超过了全局最大,更新全局
}
return global_max;
}
int main() {
int arr[] = { -2, 1, -3, 4, -1, 2, 1, -5, 4 };
int n = sizeof(arr) / sizeof(arr[0]);
int max_sum = maxSubarraySum(arr, n);
std::cout << "最大子段和: " << max_sum << std::endl;
// 记录运行时间的功能取决于使用的操作系统和库函数
// C++标准库不直接提供计时功能,可以使用chrono库或第三方库如gperftools
// 实际上测量运行时间可能会受到很多因素影响,这里简化了演示
// 使用clock()函数粗略估算,注意这只是一个示例,不是实际性能衡量
auto start = std::chrono::high_resolution_clock::now();
maxSubarraySum(arr, n);
auto end = std::chrono::high_resolution_clock::now();
long duration = std::chrono::duration_cast<std::chrono::microseconds>(end - start).count();
std::cout << "运行时间(微秒): " << duration << std::endl;
return 0;
}
```
请注意,在实际应用中,为了更准确地测量程序运行时间,通常会使用系统特定的性能分析工具或第三方库,如C++11后的`std::chrono`库或其他专门的性能分析库。上面的代码仅作为一个演示如何计算简单时间跨度的例子。
阅读全文
相关推荐
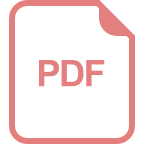
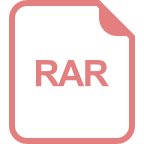
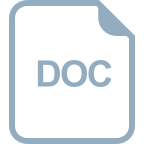
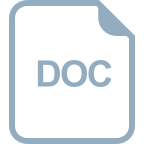
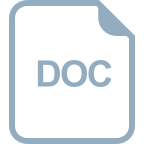
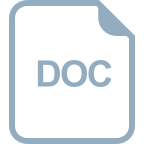
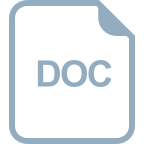
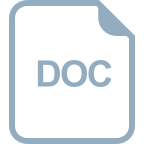
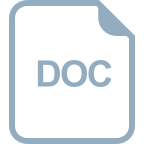
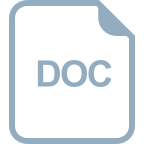
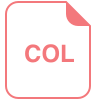
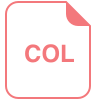
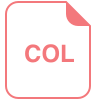
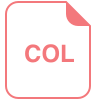
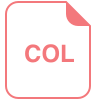

