@Autowire注解的pom依赖
时间: 2024-02-24 11:47:12 浏览: 72
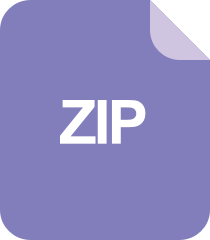
根据pom.xml下载依赖
使用@Autowired注解需要导入spring-context依赖包。在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>版本号</version>
</dependency>
```
阅读全文
相关推荐
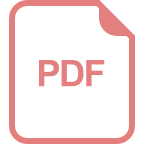
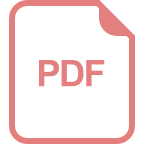
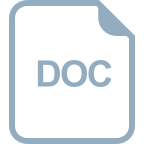














