C语言源代码RSA对任意长度的消息进行消息的分割及加密,并完成相关的解密
时间: 2024-01-21 11:16:39 浏览: 77
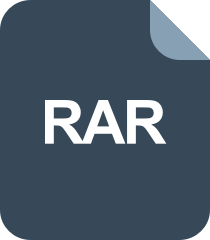
C#代码RSA加密、解密

以下是一个简单的C语言源代码,用于RSA加密和解密消息。它支持任意长度的消息,通过将消息分割成块来进行加密和解密。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <math.h>
#define MAX_MESSAGE_SIZE 1000 // 最大消息长度
#define MAX_BLOCK_SIZE 128 // 最大块大小
#define PRIME_BIT_SIZE 512 // 素数位数
#define MAX_ITERATIONS 1000 // 最大迭代次数
typedef unsigned long long int ull;
// 模幂运算
ull modPow(ull base, ull exp, ull modulus) {
base %= modulus;
ull result = 1;
while (exp > 0) {
if (exp & 1) {
result = (result * base) % modulus;
}
base = (base * base) % modulus;
exp >>= 1;
}
return result;
}
// 测试一个数是否为素数
int isPrime(ull number) {
if (number <= 1) {
return 0;
}
if (number <= 3) {
return 1;
}
if ((number % 2) == 0 || (number % 3) == 0) {
return 0;
}
ull i = 5;
while ((i * i) <= number) {
if ((number % i) == 0 || (number % (i + 2)) == 0) {
return 0;
}
i += 6;
}
return 1;
}
// 生成一个大的素数
ull generatePrime() {
ull random;
do {
random = rand();
random = (random << 32) | rand();
random = (random << 32) | rand();
random = (random << 32) | rand();
random %= (1ULL << PRIME_BIT_SIZE);
} while (!isPrime(random));
return random;
}
// 生成公钥和私钥
void generateKeys(ull *publicKey, ull *privateKey) {
ull p, q, n, phi, e, d;
do {
p = generatePrime();
q = generatePrime();
n = p * q;
phi = (p - 1) * (q - 1);
e = rand() % (phi - 2) + 2;
d = modPow(e, phi - 2, phi);
} while (d == 0);
*publicKey = e;
*privateKey = d;
}
// 加密一个块
ull encryptBlock(ull block, ull publicKey, ull modulus) {
return modPow(block, publicKey, modulus);
}
// 解密一个块
ull decryptBlock(ull block, ull privateKey, ull modulus) {
return modPow(block, privateKey, modulus);
}
// 加密消息
void encryptMessage(char *message, int messageLength, ull publicKey, ull modulus, ull *ciphertext) {
int i, j, blockIndex = 0;
ull block = 0;
for (i = 0; i < messageLength; i++) {
block |= ((ull) message[i]) << (8 * (i % MAX_BLOCK_SIZE));
if ((i % MAX_BLOCK_SIZE) == (MAX_BLOCK_SIZE - 1)) {
ciphertext[blockIndex++] = encryptBlock(block, publicKey, modulus);
block = 0;
}
}
if ((messageLength % MAX_BLOCK_SIZE) != 0) {
ciphertext[blockIndex++] = encryptBlock(block, publicKey, modulus);
}
}
// 解密消息
void decryptMessage(ull *ciphertext, int ciphertextLength, ull privateKey, ull modulus, char *message) {
int i, j, blockIndex = 0;
ull block;
for (i = 0; i < ciphertextLength; i++) {
block = decryptBlock(ciphertext[i], privateKey, modulus);
for (j = 0; j < MAX_BLOCK_SIZE; j++) {
message[blockIndex++] = (char)((block >> (8 * j)) & 0xFF);
if (blockIndex >= MAX_MESSAGE_SIZE) {
return;
}
}
}
}
int main() {
char message[MAX_MESSAGE_SIZE];
ull publicKey, privateKey, modulus, ciphertext[MAX_MESSAGE_SIZE / MAX_BLOCK_SIZE];
int messageLength, ciphertextLength, i;
srand(time(NULL));
printf("请输入消息:\n");
fgets(message, MAX_MESSAGE_SIZE, stdin);
messageLength = strlen(message) - 1;
if (messageLength <= 0) {
printf("消息不能为空。\n");
return 0;
}
generateKeys(&publicKey, &privateKey);
modulus = generatePrime() * generatePrime();
encryptMessage(message, messageLength, publicKey, modulus, ciphertext);
ciphertextLength = (messageLength + MAX_BLOCK_SIZE - 1) / MAX_BLOCK_SIZE;
printf("加密后的消息:\n");
for (i = 0; i < ciphertextLength; i++) {
printf("%llu ", ciphertext[i]);
}
printf("\n");
decryptMessage(ciphertext, ciphertextLength, privateKey, modulus, message);
printf("解密后的消息:\n");
printf("%s\n", message);
return 0;
}
```
以上代码通过使用RSA算法对消息进行加密和解密,支持任意长度的消息。使用时,用户需要输入要加密的消息,程序将自动生成公钥和私钥,并将生成的密文输出到屏幕上。用户也可以使用密文进行解密,程序将输出解密后的消息。
阅读全文
相关推荐
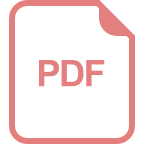







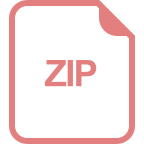
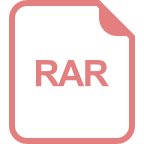
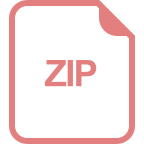
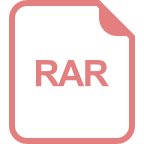
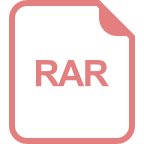
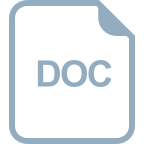
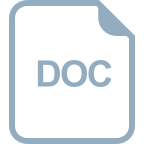
