用unity写一个冒泡函数
时间: 2024-05-03 15:17:16 浏览: 121
以下是一个用C#语言实现的冒泡排序函数:
```
void BubbleSort(int[] arr){
int n = arr.Length;
for (int i = 0; i < n-1; i++){
for (int j = 0; j < n-i-1; j++){
if (arr[j] > arr[j+1]){
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
```
该函数接受一个整型数组作为参数,对数组中的元素进行冒泡排序,即将相邻的元素进行比较,如果前一个元素大于后一个元素,就将它们交换位置,直到数组中的所有元素都按照从小到大的顺序排列好。在Unity中使用该函数时,只需要将数组作为参数传入即可。
相关问题
用unity生成一个冒泡排序
以下是用Unity生成一个冒泡排序的示例代码:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class BubbleSort : MonoBehaviour
{
public List<int> unsortedList;
public List<int> sortedList;
// Start is called before the first frame update
void Start()
{
sortedList = BubbleSortList(unsortedList);
}
List<int> BubbleSortList(List<int> inputList)
{
int n = inputList.Count;
bool swapped;
do
{
swapped = false;
for (int i = 1; i < n; i++)
{
if (inputList[i - 1] > inputList[i])
{
int temp = inputList[i - 1];
inputList[i - 1] = inputList[i];
inputList[i] = temp;
swapped = true;
}
}
n--;
} while (swapped);
return inputList;
}
}
```
该代码定义了一个BubbleSort类,其中包含两个公共列表变量:unsortedList和sortedList。在Start函数中,将unsortedList传递给BubbleSortList函数进行排序,并将结果存储在sortedList中。
BubbleSortList函数实现了冒泡排序算法,对传入的列表进行排序,并返回排序后的列表。该算法通过不断比较相邻的元素,若前一个元素大于后一个元素,则交换它们的位置,直到所有元素都被遍历一遍且没有交换发生为止。
在Unity中,可以将该脚本作为组件添加到任何游戏对象上,并将未排序的整数列表输入到unsortedList变量中。调用Start函数后,sortedList变量将包含已排序的列表。
帮我写一个Unity冒泡排序
### 回答1:
我可以为您提供简单的Unity冒泡排序示例代码:int[] arr = {5, 1, 4, 2, 8};
for (int i = 0; i < arr.Length; i++)
{
for (int j = i + 1; j < arr.Length; j++)
{
if (arr[i] > arr[j])
{
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
}
### 回答2:
冒泡排序是一种经典的排序算法,其思想是多次遍历待排序数组,每次遍历将最大的元素依次向数组末尾冒泡。下面是一个使用Unity脚本语言C#实现的冒泡排序算法:
```csharp
using UnityEngine;
public class BubbleSort : MonoBehaviour
{
void Start()
{
int[] array = { 7, 2, 9, 1, 5 };
BubbleSortAlgorithm(array);
// 输出排序结果
foreach (int element in array)
{
Debug.Log(element);
}
}
void BubbleSortAlgorithm(int[] array)
{
int length = array.Length;
for (int i = 0; i < length - 1; i++)
{
for (int j = 0; j < length - i - 1; j++)
{
// 如果当前元素大于下一个元素,进行交换
if (array[j] > array[j + 1])
{
int temp = array[j];
array[j] = array[j + 1];
array[j + 1] = temp;
}
}
}
}
}
```
在这个示例中,我们首先创建了一个包含一些整数的数组,并调用BubbleSortAlgorithm函数对其进行排序。该函数使用两个嵌套的循环遍历数组,比较相邻的元素并进行交换,直到数组排序完成。最后,我们使用foreach循环输出排序结果。
希望以上代码对你有所帮助!
### 回答3:
当然,我很愿意帮助你写一个Unity冒泡排序算法。
冒泡排序是一种简单直观的排序算法,它通过多次遍历数据,比较相邻元素的大小,并根据需要进行交换,最终使得数据按照升序或降序排列。
下面是一个简单的Unity脚本示例来实现冒泡排序:
```csharp
using UnityEngine;
public class BubbleSort : MonoBehaviour
{
void Start()
{
// 创建需要排序的整数数组
int[] array = new int[] { 5, 2, 9, 1, 3 };
// 调用冒泡排序函数对数组进行排序
BubbleSortArray(array);
// 打印排序后的结果
foreach (int num in array)
{
Debug.Log(num);
}
}
void BubbleSortArray(int[] arr)
{
int length = arr.Length;
for (int i = 0; i < length - 1; i++)
{
for (int j = 0; j < length - i - 1; j++)
{
if (arr[j] > arr[j + 1])
{
// 交换相邻元素的位置
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
}
```
在Start方法中,我们创建一个整型数组,然后调用BubbleSortArray函数对数组进行排序。BubbleSortArray函数使用两个嵌套的for循环来进行遍历和比较,并通过交换元素的方式实现排序。
最后,我们通过foreach循环打印排序后的结果。
希望这个示例能够帮助你理解和实现Unity中的冒泡排序算法。如果有任何问题,请随时向我提问。
阅读全文
相关推荐




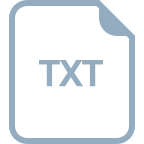
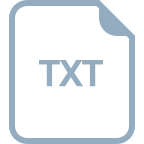
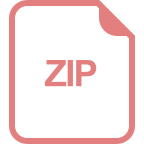
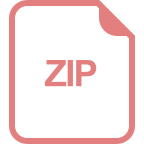
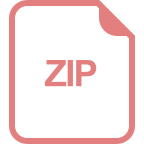
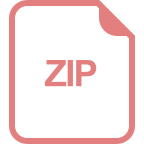
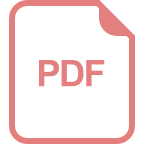
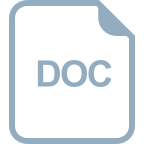
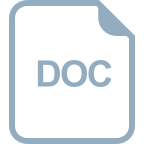
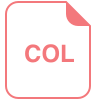