写一段用unity延时1秒的函数
时间: 2024-05-14 08:15:48 浏览: 143
好的,对于您的问题,这是一段使用Unity延迟1秒的函数:
```csharp
using System.Collections;
using UnityEngine;
public class DelayedFunction : MonoBehaviour
{
private void Start()
{
StartCoroutine(DelayedCall());
}
private IEnumerator DelayedCall()
{
yield return new WaitForSeconds(1f);
//执行您的代码或功能
}
}
```
在此代码中,我们在Start()方法中启动了一个协程,并将其传递给DelayedCall()方法。这个协程将在1秒钟后执行我们的代码或功能。请注意,我们使用了`WaitForSeconds`函数来延迟调用,这是Unity中的一种常用方法。
相关问题
写一段用unity延时1秒的程序
好的,以下是使用Unity延时1秒的程序代码:
```csharp
using UnityEngine;
using System.Collections;
public class DelayScript : MonoBehaviour
{
public float delayTime = 1.0f;
IEnumerator Start()
{
yield return new WaitForSeconds(delayTime);
Debug.Log("One second has passed.");
}
}
```
上述代码中,我们使用了协程(Coroutine)和 WaitForSeconds(等待秒数)函数来实现Unity延时1秒的效果。具体来说,Start()方法是每个MonoBehaviour派生类的内置方法,是组件启动时第一个执行的方法,它默认返回类型是void,但如果在方法中加入yield语句,则返回类型必须为IEnumerator,表示事实上暂停了一段时间,并且可以在暂停的过程中执行一些其他代码。在这个例子中,我们使用Yield Return语句暂停了一秒钟,然后再打印出一条消息。
unity延时函数
在Unity中可以使用协程(Coroutine)来实现延时函数的效果。协程是一种特殊的函数类型,可以在函数执行过程中暂停,等待一段时间后再继续执行。以下是一个使用协程实现延时函数的示例代码:
```csharp
using UnityEngine;
using System.Collections;
public class DelayExample : MonoBehaviour
{
void Start()
{
StartCoroutine(DelayedFunction(2.0f));
}
IEnumerator DelayedFunction(float delayTime)
{
yield return new WaitForSeconds(delayTime);
Debug.Log("Delayed function called after " + delayTime + " seconds");
}
}
```
在上述示例代码中,Start()函数调用了一个协程函数DelayedFunction,并传入了一个延时时间2.0f。DelayedFunction中使用yield return new WaitForSeconds(delayTime)语句来暂停函数执行,并等待指定的时间后再继续执行。在等待时间结束后,会输出一条日志信息。
除了使用协程,还可以使用Invoke函数来实现延时效果。Invoke函数可以在指定时间后调用一个指定的函数。以下是一个使用Invoke实现延时函数的示例代码:
```csharp
using UnityEngine;
public class DelayExample : MonoBehaviour
{
void Start()
{
Invoke("DelayedFunction", 2.0f);
}
void DelayedFunction()
{
Debug.Log("Delayed function called after 2 seconds");
}
}
```
在上述示例代码中,Start()函数使用Invoke函数来调用DelayedFunction,并指定了延时时间2.0f。在指定时间结束后,会调用DelayedFunction函数,并输出一条日志信息。
阅读全文
相关推荐
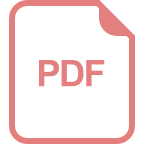




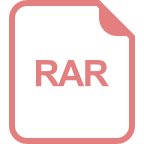



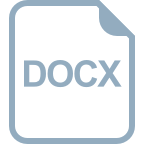
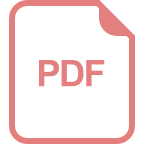
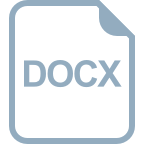