1.建立图 2.深度优先遍历 3.广度优先遍历 用C语言编写出来
时间: 2024-12-19 09:19:15 浏览: 6
1. **建立图**(创建邻接矩阵或邻接表表示)
- C语言中,我们可以使用数组或链表来构建无向图。如果使用邻接矩阵,可以定义一个二维数组,其中`matrix[i][j] = 1`表示节点i到节点j有边;如果使用邻接表,则每个节点会有一个链接列表存储其相邻节点。
```c
typedef struct Node {
int vertex;
struct Node* next;
} Node;
void createAdjacencyList(int numVertices) {
Node** adjacencyList = (Node**)malloc(sizeof(Node*) * numVertices);
for (int i = 0; i < numVertices; i++) {
adjacencyList[i] = NULL;
}
}
```
2. **深度优先搜索**(DFS)
```c
void dfs(int start, Node** adjacencyList, int visited[], int numVertices) {
visited[start] = 1;
printf("%d ", start);
Node* node = adjacencyList[start];
while (node != NULL) {
if (visited[node->vertex] == 0) {
dfs(node->vertex, adjacencyList, visited, numVertices);
}
node = node->next;
}
}
```
3. **广度优先搜索**(BFS)
```c
void bfs(int start, Node** adjacencyList, int numVertices) {
int queue[numVertices], front = 0, rear = 0;
visited[start] = 1;
queue[rear++] = start;
while (front < rear) {
int currentVertex = queue[front++];
printf("%d ", currentVertex);
Node* node = adjacencyList[currentVertex];
while (node != NULL) {
if (visited[node->vertex] == 0) {
visited[node->vertex] = 1;
queue[rear++] = node->vertex;
}
node = node->next;
}
}
}
```
阅读全文
相关推荐
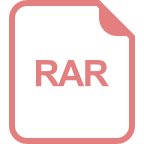
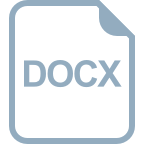
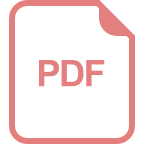















