C++使用结构体和定义模板类做一个学生成绩的线性表,具有删除,显示,查找,修改,插入,求表长等功能
时间: 2024-09-23 15:01:54 浏览: 43
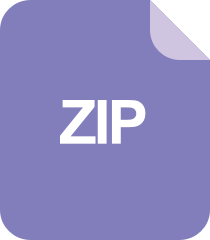
线性表和链表的存储和增删改查c++

在C++中,我们可以创建一个结构体(struct)来表示学生的成绩信息,然后定义一个模板类作为线性表,如动态数组或链表,用于存储这个学生数据结构。下面是一个简单的例子,展示如何做这样的设计:
首先,定义一个结构体`StudentScore`:
```cpp
#include <string>
// 定义学生分数结构体
struct StudentScore {
std::string name;
int score;
};
```
接着,我们定义一个模板类`LinearList`,包含基本操作功能:
```cpp
template<typename T>
class LinearList {
private:
size_t length; // 表长
T* elements; // 存储元素的指针
public:
// 构造函数
LinearList(size_t capacity = 0) : length(0), elements(new T[capacity]) {}
// 插入函数
void insert(const T& item) {
if (length == capacity) resize(capacity * 2); // 如果满则扩容
elements[length++] = item;
}
// 删除指定位置的元素
void remove(size_t index) {
if (index >= length) return;
memmove(&elements[index], &elements[index+1], sizeof(T)*(length - index - 1));
length--;
}
// 显示所有元素
void display() const {
for (size_t i = 0; i < length; ++i) {
std::cout << elements[i] << " ";
}
std::cout << "\n";
}
// 查找元素
bool find(const T& item) const {
for (size_t i = 0; i < length; ++i) {
if (elements[i] == item) return true;
}
return false;
}
// 修改元素
void modify(size_t index, const T& newItem) {
if (index < length) elements[index] = newItem;
}
// 求表长
size_t getLength() const { return length; }
// 清理内存
~LinearList() {
delete[] elements;
}
};
// 使用示例
int main() {
LinearList<StudentScore> studentList;
studentList.insert(StudentScore{"Alice", 95});
studentList.display(); // 输出: Alice 95
// ...其他操作...
return 0;
}
```
阅读全文
相关推荐
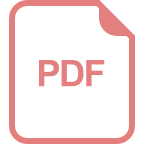
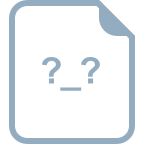
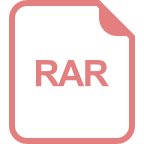
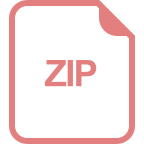
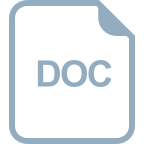
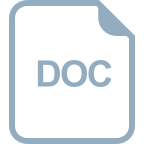


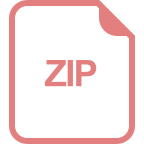
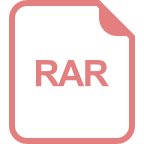
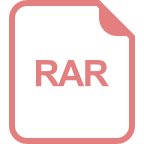
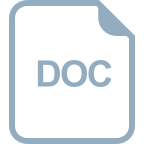
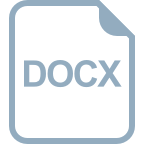
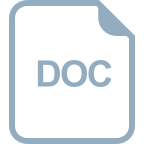
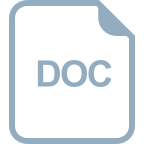
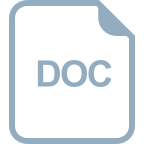
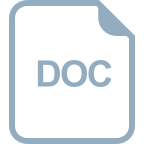
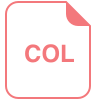