void MainWindow::sendToBack() { if (scene->selectedItems().isEmpty()) return; QGraphicsItem *selectedItem = scene->selectedItems().first(); const QList<QGraphicsItem *> overlapItems = selectedItem->collidingItems(); qreal zValue = 0; for (const QGraphicsItem *item : overlapItems) { if (item->zValue() <= zValue && item->type() == DiagramItem::Type) zValue = item->zValue() - 0.1; } selectedItem->setZValue(zValue); }含义
时间: 2024-03-28 17:38:58 浏览: 18
这是一个槽函数,用于将选中的图形项置于后面。当该函数被调用时,首先判断场景中是否有选中的图形项,如果没有则直接返回。否则,获取第一个选中的图形项,并获取与其重叠的所有图形项。然后,遍历这些重叠的图形项,找到最小的zValue值,并将选中的图形项的zValue值设置为该值减去0.1,从而使其置于重叠图形项的后面。其中,zValue值用于控制图形项的前后顺序,值越大的图形项越靠近前面。DiagramItem::Type是一个枚举值,表示图形项的类型。
相关问题
逐行解释这段代码void MainWindow::on_pushButton_clicked()//点击pushButton按钮,调用MainWindow类的槽函数 { shouldstop = 0; std::string strDataSize = ui->cbMemsize->currentText().toStdString(); std::string strFielPath = ui->lineEdit_2->text().toStdString(); std::string strFreq = ui->cbFreq->currentText().toStdString(); std::string strClock = ui->cbClk->currentData().toString().toStdString(); std::string strMode = ui->cbMode->currentData().toString().toStdString(); { int iMode = ui->cbMode->currentData().toInt(); board_channel_number = MAX_CHANNEL; if (iMode == 1 || iMode == 2) { board_channel_number = 2; } } auto pathExe = QApplication::applicationDirPath() + "/pingpong_example.exe "; std::string strPath = pathExe.toUtf8().data() + strDataSize + " " + strFielPath + " " + strFreq + " " + strClock + " " + strMode;
解释这段代码的逐行解释如下:
1. shouldstop = 0;:将变量shouldstop的值设置为0。这个变量可能是用来控制某个操作或者状态的标志。
2. std::string strDataSize = ui->cbMemsize->currentText().toStdString();:获取ui对象中名为cbMemsize的控件的当前文本内容,并将其转换为std::string类型,赋值给变量strDataSize。
3. std::string strFielPath = ui->lineEdit_2->text().toStdString();:获取ui对象中名为lineEdit_2的控件的文本内容,并将其转换为std::string类型,赋值给变量strFielPath。
4. std::string strFreq = ui->cbFreq->currentText().toStdString();:获取ui对象中名为cbFreq的控件的当前文本内容,并将其转换为std::string类型,赋值给变量strFreq。
5. std::string strClock = ui->cbClk->currentData().toString().toStdString();:获取ui对象中名为cbClk的控件的当前数据内容,并将其转换为std::string类型,赋值给变量strClock。
6. std::string strMode = ui->cbMode->currentData().toString().toStdString();:获取ui对象中名为cbMode的控件的当前数据内容,并将其转换为std::string类型,赋值给变量strMode。
7. { }:这是一个代码块,用于限定局部变量的作用域。
8. int iMode = ui->cbMode->currentData().toInt();:获取ui对象中名为cbMode的控件的当前数据内容,并将其转换为整数类型,赋值给变量iMode。
9. board_channel_number = MAX_CHANNEL;:将board_channel_number变量的值设置为MAX_CHANNEL的值。可能是用来控制通道数量的变量。
10. if (iMode == 1 || iMode == 2):如果iMode的值等于1或等于2,执行以下代码块。
11. board_channel_number = 2;:将board_channel_number变量的值设置为2。
12. auto pathExe = QApplication::applicationDirPath() + "/pingpong_example.exe ";:获取当前应用程序的路径,并将其与字符串"/pingpong_example.exe "拼接,赋值给变量pathExe。auto关键字用于自动推断变量类型。
13. std::string strPath = pathExe.toUtf8().data() + strDataSize + " " + strFielPath + " " + strFreq + " " + strClock + " " + strMode;:将pathExe、strDataSize、strFielPath、strFreq、strClock和strMode拼接成一个完整的字符串,赋值给变量strPath。这个字符串估计会被用于执行一个外部程序。
void MainWindow::iniUI() { ui->tvBook->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch); ui->tvUser->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch); ui->tvOrder->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch); ui->tvUserOrder->horizontalHeader()->setSectionResizeMode(QHeaderView::Stretch);
这段代码是用来初始化UI界面的,其中设置了四个表格的水平表头的拉伸模式为QHeaderView::Stretch,即随着窗口大小的变化,表头会自适应拉伸,保证表格数据的显示效果。其中ui->tvBook、ui->tvUser、ui->tvOrder、ui->tvUserOrder分别代表四个表格控件。如果你有关于这段代码的具体问题或疑问,可以继续提出来。
相关推荐
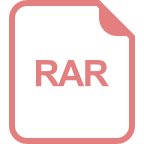
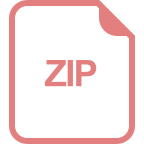









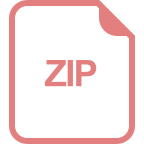
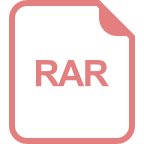
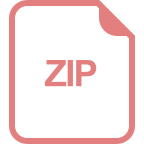
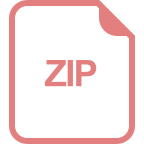