python测试计算机算力
时间: 2023-09-21 10:03:40 浏览: 155
在Python中,你可以使用time模块来测试计算机的算力。可以使用time.time()函数来获取当前时间戳,然后执行一些计算操作,最后再使用time.time()函数获取结束时间戳,计算两个时间戳之间的差值即为计算时间。示例代码如下:
```python
import time
start_time = time.time()
# 执行计算操作
result = 0
for i in range(1, 10000001):
result += i
end_time = time.time()
print("计算结果:", result)
print("计算时间:", end_time - start_time, "秒")
```
在上面的示例代码中,我们执行了一个简单的计算操作,即计算1到10000000的整数之和。我们使用time.time()函数来获取开始时间和结束时间,并计算两个时间戳之间的差值来得到计算时间。
相关问题
python测试电脑的GPU算力
要测试电脑的GPU算力,可以使用Python中的NumPy和CuPy库。
首先,需要安装CuPy库,可以使用以下命令进行安装:
```
pip install cupy
```
安装完成后,可以使用以下代码测试GPU算力:
```python
import cupy as cp
import numpy as np
# 创建一个大小为10000的随机矩阵
x = cp.random.rand(10000)
# 计算矩阵的和
sum_result = cp.sum(x)
# 将结果从GPU上复制到CPU上
print(sum_result.get())
```
这段代码会在GPU上生成一个大小为10000的随机矩阵,并计算矩阵的和。然后将结果从GPU上复制到CPU上并打印输出。运行这段代码,可以测试GPU的算力。
pythonGPU双精度算力测试代码
在Python中,如果你想要测试GPU的双精度浮点数(通常指单精度为float64)计算能力,你可以利用深度学习库如PyTorch或TensorFlow提供的功能。下面是一个简单的例子,使用PyTorch:
```python
import torch
# 检查可用的GPU设备及其CUDA版本
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
print(f"Running on {device}: CUDA version {torch.version.cuda}")
# 创建一个大的张量并将其移动到GPU上
large_tensor = torch.randn(1024, 1024).double().to(device)
start_time = torch.cuda.Event(enable_timing=True)
end_time = torch.cuda.Event(enable_timing=True)
# 执行一次双精度浮点运算,例如矩阵乘法
with torch.no_grad():
start_time.record()
result = torch.matmul(large_tensor, large_tensor.t())
end_time.record()
# 等待所有事件完成计时
torch.cuda.synchronize()
# 计算时间
time_taken = start_time.elapsed_time(end_time) / 1e3
print(f"Time taken for a double precision matrix multiplication: {time_taken:.6f} ms")
阅读全文
相关推荐
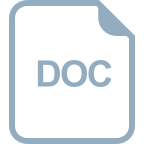
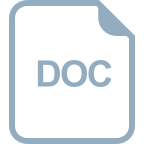
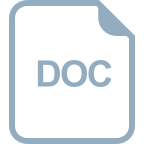
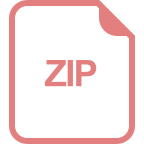
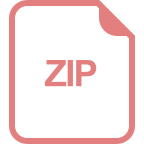






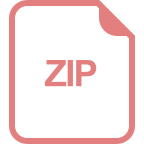
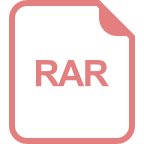
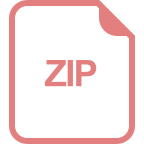
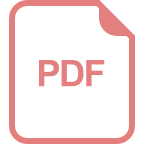
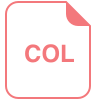
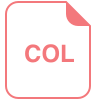