Write a javascript function,Given an array of integers, find the one that appears an odd number of times. There will always be only one integer that appears an odd number of times. Examples [7] should return 7, because it occurs 1 time (which is odd). [0] should return 0, because it occurs 1 time (which is odd). [1,1,2] should return 2, because it occurs 1 time (which is odd). [0,1,0,1,0] should return 0, because it occurs 3 times (which is odd). [1,2,2,3,3,3,4,3,3,3,2,2,1] should return 4, because it appears 1 time (which is odd).
时间: 2024-04-02 17:36:44 浏览: 28
Sure, here's a JavaScript function that solves the problem you described with the given examples:
```javascript
function findOdd(arr) {
let count = {};
for (let i = 0; i < arr.length; i++) {
if (!count[arr[i]]) {
count[arr[i]] = 1;
} else {
count[arr[i]]++;
}
}
for (let key in count) {
if (count[key] % 2 !== 0) {
return parseInt(key);
}
}
}
```
This function uses an object to store the count of each integer in the array. We then loop through the object and return the integer that appears an odd number of times.
相关问题
Write a javascript functionGiven an array of integers, find the one that appears an odd number of times. There will always be only one integer that appears an odd number of times.
Sure, here's a JavaScript function that solves the problem you described:
```javascript
function findOdd(arr) {
let result = 0;
for (let i = 0; i < arr.length; i++) {
result ^= arr[i];
}
return result;
}
```
This function uses the XOR (^) operator to find the integer that appears an odd number of times. The XOR operator returns a 1 in each bit position for which the corresponding bits of either but not both operands are 1s. By XORing all the integers in the array together, we end up with the integer that appears an odd number of times.
Given an integer array nums where every element appears three times except for one, which appears exactly once. Find the single element and return it.
To solve this problem, we can use bit manipulation. We can count the number of set bits at each position of all the integers in the array and then take the mod of this count by 3. This will give us the bit at that position in the single element.
Here's the code:
```
int singleNumber(vector<int>& nums) {
int result = 0;
for(int i=0; i<32; i++) {
int sum = 0;
for(int j=0; j<nums.size(); j++) {
if((nums[j]>>i) & 1) {
sum++;
}
}
result |= (sum % 3) << i;
}
return result;
}
```
In this code, we are iterating over all the 32 bits of an integer. For each bit position, we are counting the number of integers in the array that have a set bit at that position. We are then taking the mod of this count by 3 and setting the bit in the result integer accordingly. Finally, we are returning the result integer as the single element.
相关推荐
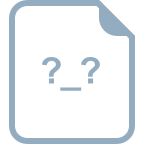
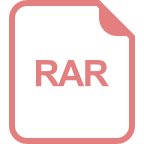
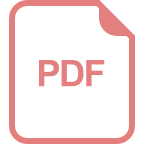












